用C语言写一个步进电机驱动程序
时间: 2023-10-04 21:12:23 浏览: 154
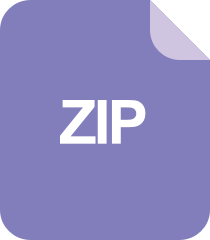
基于C语言和HAL库开发的步进电机驱动程序源码.zip
当然,我可以帮你写一个步进电机驱动程序的示例代码。以下是一个简单的步进电机驱动程序的示例,使用C语言编写:
```c
#include <stdio.h>
#include <stdlib.h>
#include <wiringPi.h>
// 定义步进电机的引脚
#define IN1 0
#define IN2 1
#define IN3 2
#define IN4 3
// 步进电机每个阶段的状态
const int steps[8][4] = {
{1, 0, 0, 0},
{1, 1, 0, 0},
{0, 1, 0, 0},
{0, 1, 1, 0},
{0, 0, 1, 0},
{0, 0, 1, 1},
{0, 0, 0, 1},
{1, 0, 0, 1}
};
// 初始化步进电机引脚
void initStepperMotor() {
wiringPiSetup();
pinMode(IN1, OUTPUT);
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
}
// 控制步进电机旋转指定的步数和方向
void rotateStepperMotor(int stepsToMove, int direction) {
int stepCount = 0;
int stepIndex = 0;
while (stepCount < stepsToMove) {
for (int pin = 0; pin < 4; pin++) {
digitalWrite(pin, steps[stepIndex][pin]);
}
delayMicroseconds(1000); // 控制步进电机速度
if (direction == 1) {
stepIndex++;
if (stepIndex > 7) {
stepIndex = 0;
}
} else {
stepIndex--;
if (stepIndex < 0) {
stepIndex = 7;
}
}
stepCount++;
}
}
int main() {
int stepsToMove = 200; // 旋转的步数
int direction = 1; // 旋转的方向(1为正转,-1为反转)
initStepperMotor();
rotateStepperMotor(stepsToMove, direction);
return 0;
}
```
请注意,此示例代码使用了wiringPi库来控制GPIO引脚。你需要在编译之前确保已经安装了该库,并将其链接到你的程序中。此外,根据你的具体硬件配置,你可能需要调整引脚定义和步进电机旋转参数。
希望这个示例能帮助到你!如果有任何问题,请随时提问。
阅读全文
相关推荐
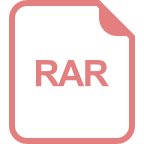


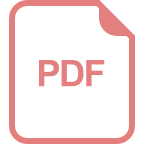
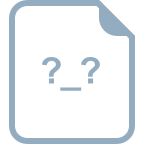
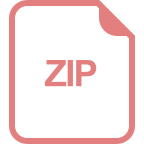
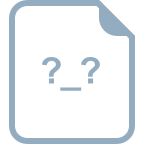
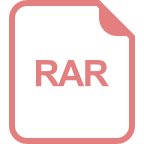



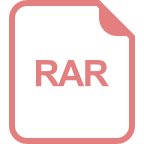
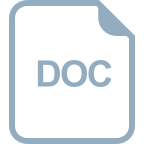
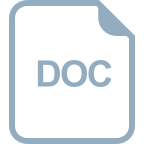
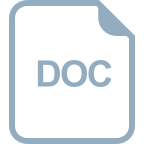
