java实现redis分布式锁
时间: 2023-06-20 08:07:15 浏览: 66
Java实现Redis分布式锁的步骤如下:
1. 首先连接Redis,使用Jedis客户端来实现,可以在pom.xml中引入以下依赖:
```xml
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.6.3</version>
</dependency>
```
2. 在Java代码中获取Redis连接:
```java
Jedis jedis = new Jedis("localhost", 6379);
```
3. 定义获取锁的方法:
```java
public boolean getLock(String key, String value, int expireTime) {
String result = jedis.set(key, value, "NX", "EX", expireTime);
return "OK".equals(result);
}
```
这里使用Redis的set命令来获取锁,其中NX表示只在键不存在时才设置值,EX表示键的过期时间为expireTime秒。
4. 定义释放锁的方法:
```java
public boolean releaseLock(String key, String value) {
String script = "if redis.call('get', KEYS[1]) == ARGV[1] then return redis.call('del', KEYS[1]) else return 0 end";
Object result = jedis.eval(script, Collections.singletonList(key), Collections.singletonList(value));
return "1".equals(result.toString());
}
```
这里使用Lua脚本来保证释放锁的原子性,如果当前锁的值与传入的值相等,则删除该键。
完整代码如下:
```java
import redis.clients.jedis.Jedis;
import java.util.Collections;
public class RedisLock {
private Jedis jedis;
public RedisLock() {
jedis = new Jedis("localhost", 6379);
}
public boolean getLock(String key, String value, int expireTime) {
String result = jedis.set(key, value, "NX", "EX", expireTime);
return "OK".equals(result);
}
public boolean releaseLock(String key, String value) {
String script = "if redis.call('get', KEYS[1]) == ARGV[1] then return redis.call('del', KEYS[1]) else return 0 end";
Object result = jedis.eval(script, Collections.singletonList(key), Collections.singletonList(value));
return "1".equals(result.toString());
}
public static void main(String[] args) {
RedisLock lock = new RedisLock();
String key = "lock_key";
String value = "lock_value";
// 获取锁
if (lock.getLock(key, value, 60)) {
System.out.println("获取锁成功");
// 业务逻辑
System.out.println("开始处理业务逻辑...");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("业务逻辑处理完成");
// 释放锁
lock.releaseLock(key, value);
System.out.println("释放锁成功");
} else {
System.out.println("获取锁失败");
}
}
}
```
相关推荐
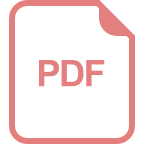
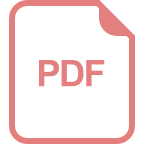
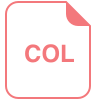
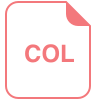
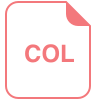
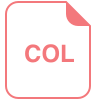
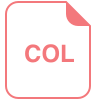









