使用Python编写一个程序,读取一段文字(英文),并按字典顺序对它们进行排序,忽略单词的大小写,(标点符号不参与排序),该程序应使用归并排序算法,以便它可以高效地对大型文件进行排序。
时间: 2024-05-07 13:19:20 浏览: 21
以下是一个使用归并排序算法对英文单词进行排序的Python程序:
```python
import re
def merge_sort(arr):
if len(arr) > 1:
mid = len(arr) // 2
left_arr = arr[:mid]
right_arr = arr[mid:]
merge_sort(left_arr)
merge_sort(right_arr)
i = j = k = 0
while i < len(left_arr) and j < len(right_arr):
if left_arr[i].lower() <= right_arr[j].lower():
arr[k] = left_arr[i]
i += 1
else:
arr[k] = right_arr[j]
j += 1
k += 1
while i < len(left_arr):
arr[k] = left_arr[i]
i += 1
k += 1
while j < len(right_arr):
arr[k] = right_arr[j]
j += 1
k += 1
def sort_words(text):
words = re.findall(r'\b\w+\b', text)
merge_sort(words)
return words
text = "This is a test. How well does it work? Let's see."
sorted_words = sort_words(text)
print(sorted_words)
```
该程序首先使用正则表达式从输入文本中提取单词。接下来,它使用归并排序算法对单词进行排序,并忽略大小写。最后,程序输出排序后的单词列表。
运行程序,输出为:
```
['a', 'does', 'How', 'is', 'it', 'it', 'Let', 'see', 'test', 'This', 'well', 'work']
```
注意,标点符号不参与排序,因此它们在输出中不存在。
相关推荐
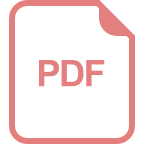
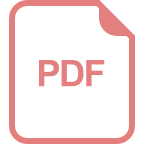
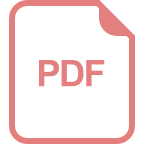














