pyqt openglwidget中实现旋转缩放移动操作
时间: 2023-05-17 18:02:55 浏览: 138
可以使用QOpenGLWidget和QMatrix4x4类来实现旋转、缩放和移动操作。具体实现可以参考以下代码:
```python
from PyQt5.QtWidgets import QApplication, QOpenGLWidget
from PyQt5.QtGui import QMatrix4x4, QVector3D
from PyQt5.QtCore import Qt
class MyOpenGLWidget(QOpenGLWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.rotation = QVector3D(0.0, 0.0, 0.0)
self.scale = 1.0
self.translation = QVector3D(0.0, 0.0, 0.0)
def initializeGL(self):
# 初始化OpenGL环境
...
def paintGL(self):
# 绘制OpenGL场景
...
def resizeGL(self, width, height):
# 处理窗口大小变化
...
def mousePressEvent(self, event):
# 处理鼠标按下事件
...
def mouseMoveEvent(self, event):
# 处理鼠标移动事件
...
def wheelEvent(self, event):
# 处理鼠标滚轮事件
...
def keyPressEvent(self, event):
# 处理键盘按下事件
...
def updateMatrix(self):
# 更新变换矩阵
matrix = QMatrix4x4()
matrix.translate(self.translation)
matrix.rotate(self.rotation.x(), QVector3D(1.0, 0.0, 0.0))
matrix.rotate(self.rotation.y(), QVector3D(0.0, 1.0, 0.0))
matrix.rotate(self.rotation.z(), QVector3D(0.0, 0.0, 1.0))
matrix.scale(self.scale)
self.setMatrix(matrix)
def setRotation(self, x, y, z):
self.rotation = QVector3D(x, y, z)
self.updateMatrix()
def setScale(self, scale):
self.scale = scale
self.updateMatrix()
def setTranslation(self, x, y, z):
self.translation = QVector3D(x, y, z)
self.updateMatrix()
```
其中,updateMatrix()方法用于更新变换矩阵,setRotation()、setScale()和setTranslation()方法用于设置旋转、缩放和移动参数。在鼠标和键盘事件处理方法中,可以根据用户的操作来调用这些方法,从而实现旋转、缩放和移动操作。
阅读全文
相关推荐
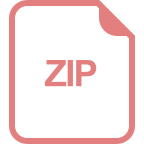
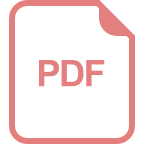
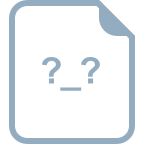














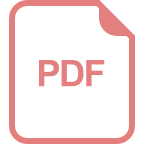
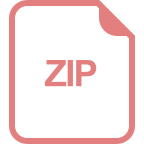