semaphore p1=0;semaphore p2,p3,p4=1; semaphore cout=0;semaphore mutex=1; void main() {P(p2);P(p3);P(4); V(cout);} write p1() {P(p1);P(metux);P(cout); 存入消息; V(p1);V(metux);} Read p2() { P(mutex);P(p1); 读消息; V(p1);V(p2);V(metux);} Read p3() { P(mutex);P(p1); 读消息; V(p1);V(p3);V(metux);} Read p4() { P(mutex);P(p1); 读消息; V(p1);V(p4); V(metux);}
时间: 2023-08-27 22:04:11 浏览: 184
这段代码是一个基于信号量的进程同步机制,实现了多个进程之间的消息传递和读取。其中,p1、p2、p3、p4是四个信号量,cout和mutex是两个互斥信号量。主函数通过对p2、p3、p4的操作,实现了消息的存入和读取。而存入消息和读取消息的函数则通过对p1、mutex、cout的操作来实现同步。其中,P()表示申请信号量,V()表示释放信号量。具体来说,这段代码实现了一种生产者-消费者模型,其中主函数扮演生产者的角色,而读取消息的函数则扮演消费者的角色。
相关问题
class Buffer { public: Buffer() { num = 0; head = 0; tail = 0; mutex = CreateSemaphore(NULL, 1, 1, NULL); semaphore_white_cell = CreateSemaphore(NULL, buffer_size, buffer_size, NULL); semaphore_black_cell = CreateSemaphore(NULL, 0, buffer_size, NULL); } ~Buffer() { CloseHandle(mutex); } bool put(int x) { WaitForSingleObject(semaphore_white_cell, INFINITE); WaitForSingleObject(mutex, INFINITE); cout << "thread " << GetCurrentThreadId() << " put " << x << "\t"; if (num == buffer_size) { cout << "failed" << endl; ReleaseSemaphore(mutex, 1, NULL); return false; } cells[tail] = x; tail = (tail + 1) % buffer_size; cout << "ok" << endl; num++; ReleaseSemaphore(mutex, 1, NULL); ReleaseSemaphore(semaphore_black_cell, 1, NULL); return true; } bool get(int* p) { WaitForSingleObject(semaphore_black_cell, INFINITE); WaitForSingleObject(mutex, INFINITE); cout << "thread" << GetCurrentThreadId() << " get\t"; if (num == 0) { cout << "failed" << endl; ReleaseSemaphore(mutex, 1, NULL); return false; } *p = cells[head]; head = (head + 1) % buffer_size; num--; cout << "ok(" << *p << ")" << endl; ReleaseSemaphore(mutex, 1, NULL); ReleaseSemaphore(semaphore_white_cell, 1, NULL); return true; } void test() { Buffer b; int i; for (i = 0; i < 7; i++) { bool ok = b.put(i); if (!ok) cout << "err: " << i << endl; } cout << "=========================\n"; int y; b.get(&y); b.get(&y); cout << "=========================\n"; for (i = 0; i < 7; i++) { int x; bool ok = b.get(&x); if (!ok) cout << "err: " << i << endl; } }
这段代码是一个使用信号量实现的缓冲区类Buffer。它具有put和get两个方法,分别用于往缓冲区中放入数据和从缓冲区中获取数据。在构造函数中,创建了三个信号量和一个互斥量。在put方法中,首先使用WaitForSingleObject函数等待semaphore_white_cell信号量,确保缓冲区中有空的单元格可以放入数据。然后使用mutex互斥量保证缓冲区的读写操作不会冲突。如果缓冲区已满,则放入数据失败,返回false;否则将数据放入缓冲区,并更新缓冲区的状态。最后释放mutex互斥量和semaphore_black_cell信号量,返回true。在get方法中,首先使用WaitForSingleObject函数等待semaphore_black_cell信号量,确保缓冲区中有数据可供获取。然后使用mutex互斥量保证缓冲区的读写操作不会冲突。如果缓冲区为空,则获取数据失败,返回false;否则获取数据并更新缓冲区的状态。最后释放mutex互斥量和semaphore_white_cell信号量,返回true。在test方法中,首先创建一个Buffer对象,然后往其中放入数据,接着从其中获取数据。最后再次从其中获取数据。
优化这段代码 #include <iostream> #include <thread> #include <chrono> #include <mutex> #include <semaphore.h> using namespace std; // shared data resource int shared_data = 0; // semaphores for synchronization sem_t mutex, rw_mutex; // number of readers int num_readers = 0; // reader function void reader(int id) { while (true) { // acquire mutex to update the number of readers sem_wait(&mutex); num_readers++; if (num_readers == 1) { // if this is the first reader, acquire the rw_mutex sem_wait(&rw_mutex); } sem_post(&mutex); // read the shared data cout << "Reader " << id << " read shared data: " << shared_data << endl; // release mutex sem_wait(&mutex); num_readers--; if (num_readers == 0) { // if this is the last reader, release the rw_mutex sem_post(&rw_mutex); } sem_post(&mutex); // sleep for a random amount of time this_thread::sleep_for(chrono::milliseconds(rand() % 1000)); } } // writer function void writer(int id) { while (true) { // acquire the rw_mutex sem_wait(&rw_mutex); // write to the shared data shared_data++; cout << "Writer " << id << " wrote to shared data: " << shared_data << endl; // release the rw_mutex sem_post(&rw_mutex); // sleep for a random amount of time this_thread::sleep_for(chrono::milliseconds(rand() % 1000)); } } int main() { // initialize semaphores sem_init(&mutex, 0, 1); sem_init(&rw_mutex, 0, 1); // create reader threads thread readers[8]; for (int i = 0; i < 8; i++) { readers[i] = thread(reader, i); } // create writer threads thread writers[2]; for (int i = 0; i < 2; i++) { writers[i] = thread(writer, i); } // join threads for (int i = 0; i < 8; i++) { readers[i].join(); } for (int i = 0; i < 2; i++) { writers[i].join(); } // destroy semaphores sem_destroy(&mutex); sem_destroy(&rw_mutex); return 0; }
这段代码是使用信号量实现读写锁的示例,但是存在一些需要优化的地方:
1. 不支持优先级反转:在这个实现中,读者和写者都使用了同一个互斥信号量(mutex)来保证对num_readers的互斥访问。但是,如果一个写者在等待互斥信号量(mutex)时,一个读者持续地获取并释放互斥信号量(mutex),那么这个写者可能会一直等待下去,从而导致优先级反转的问题。为了避免这个问题,可以使用两个互斥锁,一个用于读者,一个用于写者。
2. 读者数量计数器(num_readers)不需要互斥访问:在这个实现中,num_readers是一个全局变量,需要使用互斥信号量(mutex)来保证互斥访问。但是,实际上,这个变量只需要在读者线程中使用,因此不需要使用互斥信号量(mutex)来保护它。
3. 读者线程可能会饿死:在这个实现中,如果有一个写者在等待互斥信号量(rw_mutex),那么所有的读者都不能访问共享数据,这可能会导致读者线程饿死。为了避免这个问题,可以使用条件变量来实现读者线程的优先级。
下面是优化后的代码:
```
#include <iostream>
#include <thread>
#include <chrono>
#include <mutex>
#include <condition_variable>
#include <atomic>
#include <vector>
using namespace std;
// shared data resource
atomic<int> shared_data(0);
// mutexes for synchronization
mutex read_mutex, write_mutex;
// condition variables for synchronization
condition_variable read_cond, write_cond;
// number of readers
int num_readers = 0;
// reader function
void reader(int id)
{
while (true) {
// acquire the read_mutex to update the number of readers
unique_lock<mutex> lock(read_mutex);
num_readers++;
if (num_readers == 1) {
// if this is the first reader, acquire the write_mutex
write_mutex.lock();
}
lock.unlock();
// read the shared data
cout << "Reader " << id << " read shared data: " << shared_data << endl;
// acquire the read_mutex to update the number of readers
lock.lock();
num_readers--;
if (num_readers == 0) {
// if this is the last reader, release the write_mutex
write_mutex.unlock();
}
lock.unlock();
// sleep for a random amount of time
this_thread::sleep_for(chrono::milliseconds(rand() % 1000));
}
}
// writer function
void writer(int id)
{
while (true) {
// acquire the write_mutex
write_mutex.lock();
// write to the shared data
shared_data++;
cout << "Writer " << id << " wrote to shared data: " << shared_data << endl;
// release the write_mutex
write_mutex.unlock();
// sleep for a random amount of time
this_thread::sleep_for(chrono::milliseconds(rand() % 1000));
}
}
int main()
{
// create reader threads
vector<thread> readers(8);
for (int i = 0; i < 8; i++) {
readers[i] = thread(reader, i);
}
// create writer threads
vector<thread> writers(2);
for (int i = 0; i < 2; i++) {
writers[i] = thread(writer, i);
}
// join threads
for (int i = 0; i < 8; i++) {
readers[i].join();
}
for (int i = 0; i < 2; i++) {
writers[i].join();
}
return 0;
}
```
在这个优化后的代码中,我们使用了两个互斥锁和两个条件变量来实现读写锁。其中,read_mutex和read_cond用于保护和唤醒读者线程,write_mutex和write_cond用于保护和唤醒写者线程。通过这种方式,我们避免了优先级反转和读者线程饿死的问题。
阅读全文
相关推荐
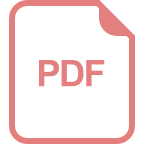
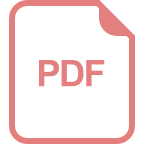
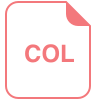
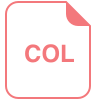








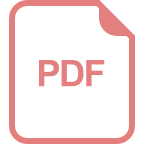
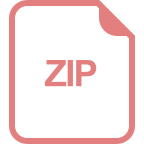
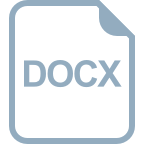