列表的合并与排序python
时间: 2024-01-22 20:02:36 浏览: 68
列表的合并可以使用 "+" 操作符或 extend() 方法实现。例如:
```python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = list1 + list2
# 或者
list1.extend(list2)
```
列表的排序可以使用 sort() 方法实现。sort() 方法默认按升序排序。例如:
```python
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
my_list.sort()
print(my_list) # [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
```
如果要按降序排序,可以指定 reverse=True 参数:
```python
my_list.sort(reverse=True)
print(my_list) # [9, 6, 5, 5, 5, 4, 3, 3, 2, 1, 1]
```
另外,还可以使用 sorted() 函数对列表进行排序,它返回一个新的已排序的列表,不改变原来的列表。例如:
```python
my_list = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
sorted_list = sorted(my_list)
print(sorted_list) # [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9]
```
相关问题
python列表的合并与排序
Python中可以使用 `+` 运算符将两个列表合并成一个新的列表。例如:
```python
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = list1 + list2
print(merged_list) # 输出 [1, 2, 3, 4, 5, 6]
```
如果要对列表进行排序,可以使用内置函数 `sorted()` 或列表的 `sort()` 方法。两者的区别在于:
- `sorted()` 函数返回一个新的排序后的列表,原列表不变;
- `sort()` 方法直接在原列表上进行排序,不返回新列表。
下面是两种排序的示例:
```python
# 使用 sorted() 函数进行排序
list1 = [3, 2, 1]
sorted_list1 = sorted(list1)
print(sorted_list1) # 输出 [1, 2, 3]
# 使用 sort() 方法进行排序
list2 = [3, 2, 1]
list2.sort()
print(list2) # 输出 [1, 2, 3]
```
需要注意的是,如果列表中的元素是字符串类型,则排序时会按照字母顺序进行排序。如果需要按照自定义规则排序,可以使用 `key` 参数传入一个函数,该函数返回每个元素的比较值。例如,下面是按照字符串长度进行排序的示例:
```python
list3 = ["abcd", "a", "bc", "defgh"]
sorted_list3 = sorted(list3, key=len)
print(sorted_list3) # 输出 ['a', 'bc', 'abcd', 'defgh']
```
python字典合并与排序
Python中可以使用update()方法将一个字典合并到另一个字典中。该方法会将待合并字典中的键值对添加到目标字典中,如果待合并字典中存在与目标字典中相同的键,则会覆盖目标字典中的键值对。示例代码如下:
```
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 3, 'c': 4}
dict1.update(dict2)
print(dict1) # 输出结果为 {'a': 1, 'b': 3, 'c': 4}
```
Python中的字典可以使用sorted()方法进行排序,但是需要注意的是,字典是无序的,只有将其转化为可排序对象后才能进行排序。通常可以使用items()方法将字典转化为元组列表,然后指定排序规则进行排序。示例代码如下:
```
dict1 = {'a': 1, 'c': 3, 'b': 2}
sorted_dict = sorted(dict1.items(), key=lambda x: x) # 按照字典键进行升序排序
print(sorted_dict) # 输出结果为 [('a', 1), ('b', 2), ('c', 3)]
```
阅读全文
相关推荐
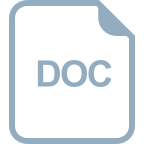














