pytorch和opencv实现全景图像拼接代码
时间: 2024-02-07 22:03:07 浏览: 224
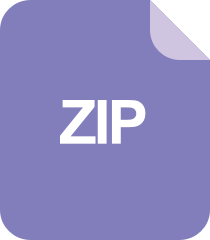
基于Python-Opencv的图片全景拼接.zip
我理解了您的问题。以下是实现全景图像拼接的Pytorch和OpenCV代码示例:
Pytorch代码示例:
```python
import torch
import cv2
import numpy as np
# 加载图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 转为pytorch tensor
img1_tensor = torch.from_numpy(img1).permute(2, 0, 1).float().unsqueeze(0)
img2_tensor = torch.from_numpy(img2).permute(2, 0, 1).float().unsqueeze(0)
# 把tensor移到GPU上,如果GPU可用
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
img1_tensor = img1_tensor.to(device)
img2_tensor = img2_tensor.to(device)
# 计算特征点和特征描述符
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 匹配特征点
bf = cv2.BFMatcher(cv2.NORM_L2, crossCheck=True)
matches = bf.match(des1, des2)
matches = sorted(matches, key=lambda x: x.distance)
# 取前几个较好的匹配点
good_matches = matches[:50]
# 计算两幅图像的投影变换矩阵
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 将两幅图像拼接起来
result = cv2.warpPerspective(img1, M, (img1.shape[1] + img2.shape[1], img2.shape[0]))
result[0:img2.shape[0], 0:img2.shape[1]] = img2
# 显示结果
cv2.imshow("Result", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
OpenCV代码示例:
```python
import cv2
import numpy as np
# 加载图像
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
# 计算特征点和特征描述符
sift = cv2.xfeatures2d.SIFT_create()
kp1, des1 = sift.detectAndCompute(img1, None)
kp2, des2 = sift.detectAndCompute(img2, None)
# 匹配特征点
bf = cv2.BFMatcher(cv2.NORM_L2, crossCheck=True)
matches = bf.match(des1, des2)
matches = sorted(matches, key=lambda x: x.distance)
# 取前几个较好的匹配点
good_matches = matches[:50]
# 计算两幅图像的投影变换矩阵
src_pts = np.float32([kp1[m.queryIdx].pt for m in good_matches]).reshape(-1, 1, 2)
dst_pts = np.float32([kp2[m.trainIdx].pt for m in good_matches]).reshape(-1, 1, 2)
M, mask = cv2.findHomography(src_pts, dst_pts, cv2.RANSAC, 5.0)
# 将两幅图像拼接起来
result = cv2.warpPerspective(img1, M, (img1.shape[1] + img2.shape[1], img2.shape[0]))
result[0:img2.shape[0], 0:img2.shape[1]] = img2
# 显示结果
cv2.imshow("Result", result)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
希望这些代码可以帮到您实现全景图像拼接!
阅读全文
相关推荐
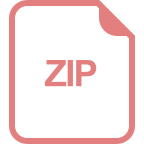
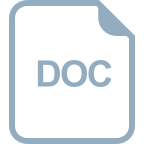















