pytorch两张特征图拼接代码怎么写
时间: 2024-03-13 13:48:08 浏览: 19
在 PyTorch 中,可以使用 `torch.cat()` 函数来拼接特征图。假设有两个特征图 `x1` 和 `x2`,它们的通道数相同,要将它们在通道维度上拼接,代码如下:
```
import torch
# 假设 x1 和 x2 的形状都为 (batch_size, channel, height, width)
x_cat = torch.cat([x1, x2], dim=1) # 在通道维度上拼接
```
其中 `dim=1` 表示在通道维度上拼接,`x_cat` 即为拼接后的特征图。若两个特征图的大小不同,需要先使用相应的操作将它们调整为相同大小再进行拼接。
相关问题
pytorch两张大小相同通道数不同的特征图拼接代码怎么写
如果两个特征图大小相同但通道数不同,可以先通过卷积操作将通道数调整为相同,再使用 `torch.cat()` 函数进行拼接。具体代码如下:
```
import torch.nn as nn
import torch
# 假设 x1 和 x2 的形状都为 (batch_size, channel1, height, width)
# channel1 和 channel2 不相等
conv = nn.Conv2d(channel1, channel2, kernel_size=1) # 定义一个 1x1 卷积调整通道数
x1_conv = conv(x1) # 将 x1 调整为 channel2 通道数
x_cat = torch.cat([x1_conv, x2], dim=1) # 在通道维度上拼接
```
其中,`nn.Conv2d()` 函数定义了一个 1x1 卷积操作,将 `x1` 调整为 `channel2` 通道数,然后使用 `torch.cat()` 函数对 `x1_conv` 和 `x2` 进行在通道维度上的拼接。
请帮我写一个pytorch实现两张图片全景图像拼接的代码 我需要在代码中导入两张图篇并且拼接结果会显示出来
好的,以下是一个简单的 pytorch 实现两张图片全景图像拼接的代码示例:
``` python
import torch
from torch.autograd import Variable
import torch.nn.functional as F
from PIL import Image
# 读取两个图像
img1 = Image.open('img1.png')
img2 = Image.open('img2.png')
# 转换为 PyTorch tensor 格式,并扩展维度
img1_tensor = Variable(F.to_tensor(img1)).unsqueeze(0)
img2_tensor = Variable(F.to_tensor(img2)).unsqueeze(0)
# 将两个图像连接到同一维度
concat_tensor = torch.cat((img1_tensor, img2_tensor), 3)
# 将连接后的图像转换为 PIL.Image 格式,并保存
concat_image = Image.fromarray(concat_tensor.squeeze().mul(255).clamp(0, 255).byte().permute(1, 2, 0).cpu().numpy())
concat_image.save('concat_image.png')
```
执行代码后,会把两张图片(img1.png 和 img2.png)连接起来并保存为一张新的图片(concat_image.png)。
注:需要将两张图片放在同一文件夹下执行代码,并根据实际需要更改图片名称。
相关推荐
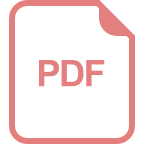
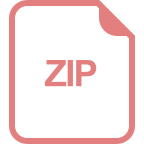











