使用 Vivado HLS 工具 通过 3 线-8 线译码器用模块化建模的方式实现 4 线-16 线译码器的功能,写出源文件和激励文件
时间: 2024-02-15 19:05:07 浏览: 92
好的,我明白了。以下是使用 Vivado HLS 工具通过 3 线-8 线译码器用模块化建模的方式实现 4 线-16 线译码器的源文件和激励文件。
源文件:
```c++
#include <ap_int.h>
void decoder_3_8(ap_uint<3> input, ap_uint<8>& output) {
#pragma HLS INTERFACE ap_ctrl_none port=return
#pragma HLS INTERFACE ap_none port=input
#pragma HLS INTERFACE ap_none port=output
ap_uint<8> temp = 0;
switch(input) {
case 0:
temp = 0x01;
break;
case 1:
temp = 0x02;
break;
case 2:
temp = 0x04;
break;
case 3:
temp = 0x08;
break;
case 4:
temp = 0x10;
break;
case 5:
temp = 0x20;
break;
case 6:
temp = 0x40;
break;
case 7:
temp = 0x80;
break;
}
output = temp;
}
void decoder_4_16(ap_uint<4> input, ap_uint<16>& output) {
#pragma HLS INTERFACE ap_ctrl_none port=return
#pragma HLS INTERFACE ap_none port=input
#pragma HLS INTERFACE ap_none port=output
ap_uint<8> low = 0;
ap_uint<8> high = 0;
decoder_3_8(input.range(2, 0), low);
decoder_3_8(input.range(3, 3), high);
output = (high, low);
}
```
激励文件:
```c++
#include <ap_int.h>
#include <iostream>
void decoder_4_16(ap_uint<4> input, ap_uint<16>& output);
int main() {
ap_uint<4> input;
ap_uint<16> output;
input = 0;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 1;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 2;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 3;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 4;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 5;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 6;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 7;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 8;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 9;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 10;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 11;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 12;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 13;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 14;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
input = 15;
decoder_4_16(input, output);
std::cout << "Input: " << input << ", Output: " << output << std::endl;
return 0;
}
```
阅读全文
相关推荐
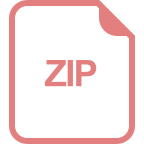


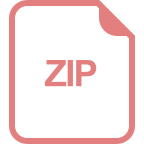
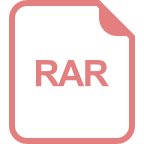
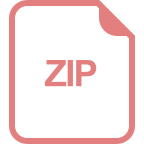
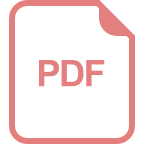
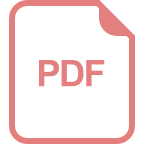
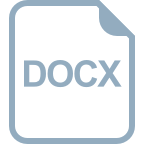
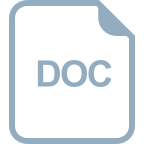
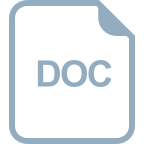
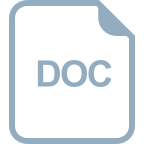
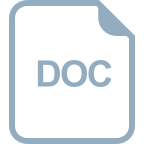
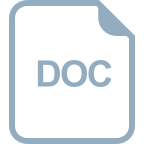
