使用hbase Java api完成以下操作: 1.建表userfollower 2.用户Mary有一个粉丝Alice,Alice关注列表中也存在Mary 3.用户Jack有两个粉丝Bob和Ben 4.Jack还关注了Mary 5.查看Jack被哪些人关注,Jack关注了谁 6.Bob取关了Jack 7.扫描全表数据 导入以下包完成import org.apache.hadoop.conf.Configuration;import org.apache.hadoop.hbase.;import org.apache.hadoop.hbase.client.;import org.apache.hadoop.hbase.util.Bytes;import java.io.IOException;
时间: 2023-08-05 22:06:32 浏览: 51
下面是使用HBase Java API完成的代码:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.*;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
import java.io.IOException;
public class HBaseExample {
public static void main(String[] args) throws IOException {
Configuration conf = HBaseConfiguration.create();
Connection connection = ConnectionFactory.createConnection(conf);
Admin admin = connection.getAdmin();
// 1.建表userfollower
TableName tableName = TableName.valueOf("userfollower");
if (!admin.tableExists(tableName)) {
TableDescriptor tableDescriptor = TableDescriptorBuilder.newBuilder(tableName)
.setColumnFamily(ColumnFamilyDescriptorBuilder.of("follower"))
.setColumnFamily(ColumnFamilyDescriptorBuilder.of("following"))
.build();
admin.createTable(tableDescriptor);
}
Table table = connection.getTable(tableName);
// 2.用户Mary有一个粉丝Alice,Alice关注列表中也存在Mary
String maryRowKey = "mary";
String aliceRowKey = "alice";
Put put = new Put(Bytes.toBytes(maryRowKey));
put.addColumn(Bytes.toBytes("follower"), Bytes.toBytes(aliceRowKey), Bytes.toBytes("follow"));
put.addColumn(Bytes.toBytes("following"), Bytes.toBytes(aliceRowKey), Bytes.toBytes("follow"));
table.put(put);
// 3.用户Jack有两个粉丝Bob和Ben
String jackRowKey = "jack";
String bobRowKey = "bob";
String benRowKey = "ben";
put = new Put(Bytes.toBytes(jackRowKey));
put.addColumn(Bytes.toBytes("follower"), Bytes.toBytes(bobRowKey), Bytes.toBytes("follow"));
put.addColumn(Bytes.toBytes("follower"), Bytes.toBytes(benRowKey), Bytes.toBytes("follow"));
table.put(put);
// 4.Jack还关注了Mary
put = new Put(Bytes.toBytes(jackRowKey));
put.addColumn(Bytes.toBytes("following"), Bytes.toBytes(maryRowKey), Bytes.toBytes("follow"));
table.put(put);
// 5.查看Jack被哪些人关注,Jack关注了谁
Get get = new Get(Bytes.toBytes(jackRowKey));
Result result = table.get(get);
System.out.println("Jack被哪些人关注:");
for (Cell cell : result.getColumnCells(Bytes.toBytes("follower"), null)) {
System.out.println(Bytes.toString(CellUtil.cloneQualifier(cell)));
}
System.out.println("Jack关注了谁:");
for (Cell cell : result.getColumnCells(Bytes.toBytes("following"), null)) {
System.out.println(Bytes.toString(CellUtil.cloneQualifier(cell)));
}
// 6.Bob取关了Jack
Delete delete = new Delete(Bytes.toBytes(bobRowKey));
delete.addColumns(Bytes.toBytes("follower"), Bytes.toBytes(jackRowKey));
table.delete(delete);
// 7.扫描全表数据
Scan scan = new Scan();
ResultScanner scanner = table.getScanner(scan);
for (Result r : scanner) {
System.out.println("Scan: " + r);
}
table.close();
admin.close();
connection.close();
}
}
```
注意:在运行该程序之前,需要先启动HBase服务。
相关推荐
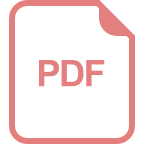





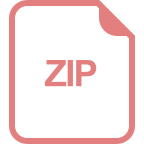
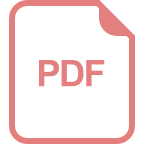
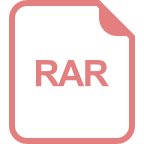
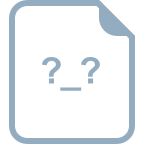
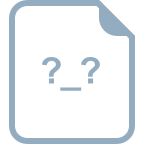
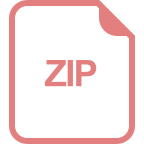
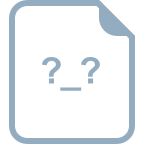