用java实现连连看小程序,连接题材用蔬菜水果的代码
时间: 2024-03-03 15:51:46 浏览: 16
好的,以下是用Java实现连连看小程序,连接题材用蔬菜水果的简单示例代码:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class ConnectFruits extends JFrame implements ActionListener {
private JButton[][] buttons; // 按钮数组
private ImageIcon[] icons; // 图标数组
private int[][] matrix; // 矩阵数组
private int rows; // 行数
private int cols; // 列数
private int currentX; // 当前选中的水果或蔬菜的横坐标
private int currentY; // 当前选中的水果或蔬菜的纵坐标
public ConnectFruits(int rows, int cols) {
this.rows = rows;
this.cols = cols;
setTitle("Connect Fruits");
setResizable(false);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel(new GridLayout(rows, cols));
buttons = new JButton[rows][cols];
icons = new ImageIcon[16];
matrix = new int[rows][cols];
for (int i = 0; i < icons.length; i++) {
icons[i] = new ImageIcon(getClass().getResource("fruit" + i + ".png"));
}
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
buttons[i][j] = new JButton();
buttons[i][j].setPreferredSize(new Dimension(64, 64));
buttons[i][j].addActionListener(this);
panel.add(buttons[i][j]);
}
}
initMatrix();
getContentPane().add(panel);
pack();
setLocationRelativeTo(null);
setVisible(true);
}
private void initMatrix() {
int count = rows * cols / 8;
int[] positions = new int[count * 2];
for (int i = 0; i < count; i++) {
int x = (int)(Math.random() * rows);
int y = (int)(Math.random() * cols);
while (matrix[x][y] != 0) {
x = (int)(Math.random() * rows);
y = (int)(Math.random() * cols);
}
matrix[x][y] = i + 1;
matrix[x][cols - y - 1] = i + 1;
positions[i * 2] = x;
positions[i * 2 + 1] = y;
}
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (matrix[i][j] == 0) {
int index = (int)(Math.random() * 16);
matrix[i][j] = index + 1;
}
buttons[i][j].setIcon(icons[matrix[i][j] - 1]);
}
}
}
private boolean hasPath(int x1, int y1, int x2, int y2) {
if (x1 == x2 && y1 == y2) return false;
if (matrix[x1][y1] != matrix[x2][y2]) return false;
if (x1 == x2) {
int min = Math.min(y1, y2);
int max = Math.max(y1, y2);
for (int j = min + 1; j < max; j++) {
if (matrix[x1][j] != 0) {
return false;
}
}
return true;
}
if (y1 == y2) {
int min = Math.min(x1, x2);
int max = Math.max(x1, x2);
for (int i = min + 1; i < max; i++) {
if (matrix[i][y1] != 0) {
return false;
}
}
return true;
}
int dx = Math.abs(x1 - x2);
int dy = Math.abs(y1 - y2);
if (dx + dy > 3) return false;
if (dx == 1 && dy == 1) {
if (matrix[x1][y2] == 0 && matrix[x2][y1] == 0) {
return true;
} else {
return false;
}
}
if (dx == 2 || dy == 2) {
return false;
}
if (dx == 1) {
int sy = (y1 + y2) / 2;
if (matrix[x1][sy] == 0 && hasPath(x1, y1, x1, sy) && hasPath(x1, sy, x2, y2)) {
return true;
}
} else {
int sx = (x1 + x2) / 2;
if (matrix[sx][y1] == 0 && hasPath(x1, y1, sx, y1) && hasPath(sx, y1, x2, y2)) {
return true;
}
}
return false;
}
private void remove(int x1, int y1, int x2, int y2) {
matrix[x1][y1] = 0;
matrix[x2][y2] = 0;
buttons[x1][y1].setIcon(null);
buttons[x2][y2].setIcon(null);
}
private boolean check() {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (matrix[i][j] != 0) {
for (int k = i + 1; k < rows; k++) {
for (int l = 0; l < cols; l++) {
if (matrix[k][l] != 0 && hasPath(i, j, k, l)) {
return false;
}
}
}
}
}
}
return true;
}
public void actionPerformed(ActionEvent e) {
JButton source = (JButton)e.getSource();
int x = -1;
int y = -1;
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
if (buttons[i][j] == source) {
x = i;
y = j;
break;
}
}
}
if (x == -1 || y == -1) return;
if (currentX == -1 && currentY == -1) {
currentX = x;
currentY = y;
source.setBorder(BorderFactory.createLineBorder(Color.YELLOW, 3));
} else {
if (hasPath(currentX, currentY, x, y)) {
remove(currentX, currentY, x, y);
if (check()) {
JOptionPane.showMessageDialog(this, "You Win!");
dispose();
}
} else {
buttons[currentX][currentY].setBorder(null);
}
currentX = -1;
currentY = -1;
}
}
public static void main(String[] args) {
new ConnectFruits(8, 8);
}
}
```
这段代码实现了一个8x8的连连看小游戏,其中用16张不同的水果或蔬菜图片来填充矩阵,每种图片各有两个。玩家需要通过点击图片来消除相同的两个水果或蔬菜,直到全部消除完毕为止。
相关推荐
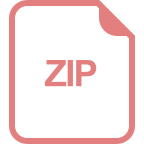














