写一个实现二维离散傅里叶变换的C语言代码
时间: 2024-05-10 13:21:31 浏览: 9
以下是一个实现二维离散傅里叶变换的C语言代码的示例:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
// 复数结构体
typedef struct {
double re; // 实部
double im; // 虚部
} complex;
// 二维离散傅里叶变换函数
void dft2d(complex **input, complex **output, int width, int height) {
int i, j, u, v;
double arg;
complex sum;
// 外层循环遍历输出矩阵的每个元素
for (u = 0; u < height; u++) {
for (v = 0; v < width; v++) {
sum.re = 0.0;
sum.im = 0.0;
// 内层循环计算傅里叶变换公式
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
arg = 2 * PI * (i * u / (double)height + j * v / (double)width);
sum.re += input[i][j].re * cos(arg) + input[i][j].im * sin(arg);
sum.im += -input[i][j].re * sin(arg) + input[i][j].im * cos(arg);
}
}
output[u][v] = sum;
}
}
}
int main() {
int i, j, width, height;
complex **input, **output;
// 读入矩阵尺寸
printf("Enter the width and height of the matrix: ");
scanf("%d %d", &width, &height);
// 动态分配数组内存
input = (complex **)malloc(height * sizeof(complex *));
output = (complex **)malloc(height * sizeof(complex *));
for (i = 0; i < height; i++) {
input[i] = (complex *)malloc(width * sizeof(complex));
output[i] = (complex *)malloc(width * sizeof(complex));
}
// 读入输入矩阵
printf("Enter the matrix values:\n");
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
scanf("%lf", &input[i][j].re);
input[i][j].im = 0.0;
}
}
// 调用二维离散傅里叶变换函数
dft2d(input, output, width, height);
// 输出结果
printf("The DFT result is:\n");
for (i = 0; i < height; i++) {
for (j = 0; j < width; j++) {
printf("(%lf, %lf) ", output[i][j].re, output[i][j].im);
}
printf("\n");
}
// 释放数组内存
for (i = 0; i < height; i++) {
free(input[i]);
free(output[i]);
}
free(input);
free(output);
return 0;
}
```
该代码中使用了一个复数结构体来表示复数,以便进行复数运算。在二维离散傅里叶变换函数中,外层循环遍历输出矩阵的每个元素,内层循环计算傅里叶变换公式。最后,在主函数中动态分配输入和输出矩阵的内存,并调用二维离散傅里叶变换函数进行计算,最后输出结果并释放内存。
相关推荐
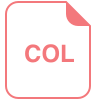
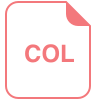
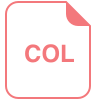
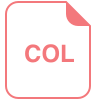










