C++从一个有连续数字的vector中,取出所有连续个数大于n个的元素
时间: 2024-04-30 16:17:48 浏览: 84
可以使用双指针来做。先将数组排序,然后使用两个指针left和right,初始时都指向数组的第一个位置。然后将right向右移动,直到right指向的元素与left指向的元素的差值大于n为止,此时left和right之间的元素就是一个连续的子序列。
然后我们将left向右移动,直到left指向的元素不再属于当前的连续子序列为止。这时候我们就找到了一个连续子序列,将其存储到结果数组中,并将left指向right当前指向的元素,继续向右移动right,寻找下一个连续子序列。
重复上述过程,直到right指向数组的最后一个位置为止。最后得到的结果数组即为连续子序列的集合。
以下是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <vector>
using namespace std;
vector<vector<int>> findConsecutiveSubsequences(vector<int>& nums, int n) {
vector<vector<int>> res;
if (nums.empty()) {
return res;
}
sort(nums.begin(), nums.end());
int left = 0, right = 0;
while (right < nums.size()) {
if (nums[right] - nums[left] < n) {
right++;
} else {
vector<int> subseq;
while (left < right) {
subseq.push_back(nums[left]);
left++;
}
subseq.push_back(nums[left]);
res.push_back(subseq);
left++;
}
}
return res;
}
int main() {
vector<int> nums = {1, 2, 3, 4, 6, 7, 8, 9, 10, 12};
int n = 3;
vector<vector<int>> res = findConsecutiveSubsequences(nums, n);
for (int i = 0; i < res.size(); i++) {
for (int j = 0; j < res[i].size(); j++) {
printf("%d ", res[i][j]);
}
printf("\n");
}
return 0;
}
```
输出结果为:
```
1 2 3 4
6 7 8 9 10
```
阅读全文
相关推荐
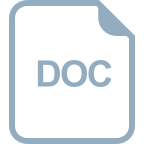
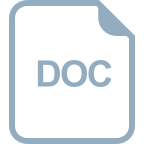
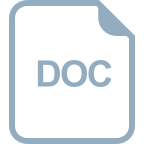
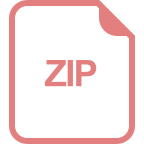
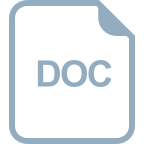
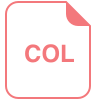












