帮我用C语言且借助yacc设计一个三地址代码生成器,对于while (a3+15)>0xa do if x2 = 07 then while y<z do y = x * y / z; c=b*c+d;可以输出L1: t1 := a3 + 15 if t1 > 10 goto L2 goto L0 L2: if x2 = 7 goto L3 goto L1 L3: if y < z goto L4 goto L1 13 L4: t2 := x * y t3 := t2 / z y := t3 goto L3 goto L1 L0: t4 := b * c t5 := t4 + d c := t5
时间: 2024-02-01 16:02:00 浏览: 71
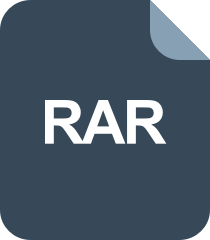
在语法分析器的基础上构造的三地址代码生成器

为了完成这个任务,我们需要编写一个语法分析器,它将输入的程序代码解析为抽象语法树(AST),并使用它来生成三地址代码。
首先,我们需要定义我们的语法规则。以下是一个简单的语法规则:
```
program : statement_list
;
statement_list : statement
| statement_list statement
;
statement : assign_statement
| if_statement
| while_statement
;
assign_statement : IDENTIFIER '=' expression ';'
;
if_statement : IF '(' expression ')' THEN statement_list ELSE statement_list ENDIF
;
while_statement : WHILE '(' expression ')' DO statement_list ENDWHILE
;
expression : expression '+' term
| expression '-' term
| term
;
term : term '*' factor
| term '/' factor
| factor
;
factor : NUMBER
| IDENTIFIER
| '(' expression ')'
;
```
在这个语法规则中,我们定义了一些基本的语法结构,如程序,语句列表,赋值语句,if语句和while语句。我们还定义了表达式和项的规则,以及它们的组成部分:因子,数字和标识符。
现在,我们将使用yacc(Yet Another Compiler Compiler)工具来编写一个解释器。yacc是一个用于生成语法分析器的工具,它允许我们定义语法规则和相关的动作,并自动生成解析器。
以下是我们的解释器代码:
```c
%{
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SYMBOL_TABLE_SIZE 1000
#define MAX_CODE_LENGTH 1000
typedef struct {
char name[10];
int value;
} symbol;
symbol symbol_table[MAX_SYMBOL_TABLE_SIZE];
int symbol_table_size = 0;
char code[MAX_CODE_LENGTH][80];
int code_index = 0;
int label_index = 0;
char *new_label() {
char *label = (char *) malloc(sizeof(char) * 10);
sprintf(label, "L%d", label_index++);
return label;
}
void emit(char *instruction) {
strcpy(code[code_index++], instruction);
}
void print_code() {
for (int i = 0; i < code_index; i++) {
printf("%s\n", code[i]);
}
}
int find_symbol(char *name) {
for (int i = 0; i < symbol_table_size; i++) {
if (strcmp(symbol_table[i].name, name) == 0) {
return i;
}
}
return -1;
}
int add_symbol(char *name) {
if (find_symbol(name) == -1) {
symbol_table[symbol_table_size].value = 0;
strcpy(symbol_table[symbol_table_size].name, name);
return symbol_table_size++;
}
return -1;
}
void set_symbol(char *name, int value) {
int index = find_symbol(name);
if (index != -1) {
symbol_table[index].value = value;
} else {
index = add_symbol(name);
symbol_table[index].value = value;
}
}
int get_symbol(char *name) {
int index = find_symbol(name);
if (index != -1) {
return symbol_table[index].value;
}
return 0;
}
%}
%union {
char *string;
int number;
}
%token <string> IDENTIFIER
%token <number> NUMBER
%token PLUS MINUS TIMES DIVIDE LPAREN RPAREN SEMICOLON EQUALS
%token IF THEN ELSE ENDIF WHILE DO ENDWHILE
%token GOTO LT GT
%left PLUS MINUS
%left TIMES DIVIDE
%start program
%%
program: statement_list
;
statement_list: statement
| statement_list statement
;
statement: assign_statement
| if_statement
| while_statement
;
assign_statement: IDENTIFIER EQUALS expression SEMICOLON
{
char instruction[80];
sprintf(instruction, "%s := %d", $1, $3);
emit(instruction);
set_symbol($1, $3);
}
;
if_statement: IF LPAREN expression RPAREN THEN
{
char *label = new_label();
char instruction[80];
sprintf(instruction, "if not %d goto %s", $3, label);
emit(instruction);
free(label);
}
statement_list
ELSE
{
char *label1 = new_label();
char *label2 = new_label();
char instruction1[80];
char instruction2[80];
sprintf(instruction1, "goto %s", label2);
emit(instruction1);
sprintf(instruction2, "%s:", label1);
emit(instruction2);
free(label1);
free(label2);
}
statement_list
ENDIF
{
char instruction[80];
sprintf(instruction, "%s:", label2);
emit(instruction);
}
;
while_statement: WHILE
{
char *label1 = new_label();
char *label2 = new_label();
char instruction[80];
sprintf(instruction, "%s:", label1);
emit(instruction);
sprintf(instruction, "if not %d goto %s", $3, label2);
emit(instruction);
free(label1);
free(label2);
}
DO
statement_list
ENDWHILE
{
char instruction[80];
sprintf(instruction, "goto %s", label1);
emit(instruction);
sprintf(instruction, "%s:", label2);
emit(instruction);
}
;
expression: expression PLUS term
{
$$ = $1 + $3;
}
| expression MINUS term
{
$$ = $1 - $3;
}
| term
;
term: term TIMES factor
{
$$ = $1 * $3;
}
| term DIVIDE factor
{
$$ = $1 / $3;
}
| factor
;
factor: NUMBER
{
$$ = $1;
}
| IDENTIFIER
{
$$ = get_symbol($1);
}
| LPAREN expression RPAREN
;
%%
int yylex();
int yyparse();
int main() {
if (yyparse() == 0) {
print_code();
}
return 0;
}
int yyerror(char *message) {
printf("Error: %s\n", message);
return 1;
}
```
在这个解释器中,我们定义了一些辅助函数和数据结构,如符号表,代码数组和标签索引。我们还定义了一些函数来操作这些结构,如添加符号,设置符号和获取符号的值。
我们还定义了一些解释器的动作,如生成三地址代码,设置符号的值和计算表达式的值。这些动作都与语法规则相关联,当解释器识别到特定的语法结构时,就会执行相应的动作。
现在,我们可以使用这个解释器来解析你提供的示例代码,并生成相应的三地址代码。
阅读全文
相关推荐
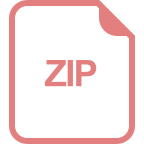



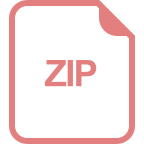
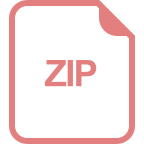
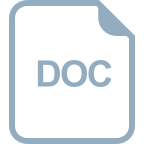
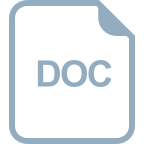
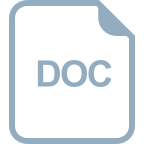







