批量导入pd到excel的sheet,使用 result_data = pd.DataFrame.from_records(df) result_data.to_excel(writer,sheet_name=sheet,index=False)时,实现每个sheet都有两个pd需要导入
时间: 2024-02-25 09:51:35 浏览: 29
你可以将两个pd合并成一个,然后再导入到一个sheet中。具体操作如下:
1. 将两个pd按照需要的方式合并成一个pd,比如使用concat()函数。
2. 将合并后的pd导入到一个sheet中,可以将sheet_name参数设置为需要导入的sheet名称。
下面是一个示例代码:
```python
import pandas as pd
# 假设有两个需要导入的pd,分别为df1和df2
df1 = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
df2 = pd.DataFrame({'A': [7, 8, 9], 'B': [10, 11, 12]})
# 将两个pd合并成一个pd
merged_df = pd.concat([df1, df2])
# 导入到excel中的sheet名称
sheet_name = 'my_sheet'
# 将合并后的pd导入到excel中
with pd.ExcelWriter('my_excel.xlsx') as writer:
merged_df.to_excel(writer, sheet_name=sheet_name, index=False)
```
这样,你就可以将两个pd合并成一个,并将其导入到指定的sheet中了。
相关问题
df = pd.read_sql_query(sql,engine) result_data = pd.DataFrame.from_records(df)中sql语句使用CALL 存储过程如何获取的返回的第三个数据集?
如果存储过程返回了多个数据集,可以使用`pymysql`模块的`nextset()`方法来获取下一个数据集。例如,以下代码演示了如何使用`pymysql`获取存储过程返回的第三个数据集:
```python
import pymysql
import pandas as pd
# 连接数据库
conn = pymysql.connect(host='localhost', user='root', password='123456', database='test')
# 创建游标对象
cursor = conn.cursor()
# 调用存储过程
cursor.callproc('your_stored_procedure')
# 获取第一个数据集
result1 = cursor.fetchall()
# 移动到下一个数据集
cursor.nextset()
# 获取第二个数据集
result2 = cursor.fetchall()
# 移动到下一个数据集
cursor.nextset()
# 获取第三个数据集
result3 = cursor.fetchall()
# 关闭游标和连接
cursor.close()
conn.close()
# 转换为DataFrame
df = pd.DataFrame.from_records(result3)
```
上述代码中,我们使用`cursor.callproc`方法调用存储过程,在获取第一个数据集后,使用`cursor.nextset()`方法移动到下一个数据集,以此类推,直到获取第三个数据集。最后将结果转换为DataFrame即可。
需要注意的是,如果存储过程返回的多个数据集中有空数据集,则需要使用`cursor.nextset()`方法跳过空数据集,否则会导致程序卡住。
df = pd.read_sql_query(sql,engine) result_data = pd.DataFrame.from_records(df)中sql语句是否可以使用CALL 存储过程的返回数据集?
是的,可以使用CALL存储过程的返回数据集。只需要将存储过程的调用语句写在sql参数里即可。例如:
```sql
CALL your_stored_procedure();
```
然后使用`pd.read_sql_query`方法读取即可。注意,存储过程返回的字段名可能与表的字段名不一致,需要根据实际情况进行调整。
相关推荐
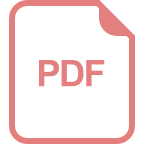
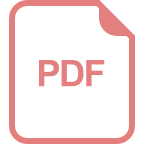
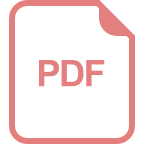












