c++计算三维点云数据最小路径源代码
时间: 2023-06-27 16:08:33 浏览: 107
以下是使用C++计算三维点云数据最小路径的示例源代码:
```c++
#include <iostream>
#include <vector>
#include <cmath>
#include <algorithm>
struct Point3D {
double x, y, z;
};
double distance(const Point3D& p1, const Point3D& p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
double dz = p1.z - p2.z;
return std::sqrt(dx*dx + dy*dy + dz*dz);
}
double minPath(const std::vector<Point3D>& points) {
int n = points.size();
std::vector<double> dp(n, 1e9);
dp[0] = 0;
for (int i = 1; i < n; i++) {
for (int j = 0; j < i; j++) {
double d = distance(points[i], points[j]);
dp[i] = std::min(dp[i], dp[j] + d);
}
}
return dp[n-1];
}
int main() {
std::vector<Point3D> points = {{0,0,0}, {1,1,1}, {2,2,2}, {3,3,3}, {4,4,4}};
double result = minPath(points);
std::cout << "Minimum path distance: " << result << std::endl;
return 0;
}
```
在上面的示例中,我们定义了一个名为`Point3D`的结构体,用于表示三维空间中的点。我们还定义了一个名为`distance`的函数来计算两个点之间的距离。最后,我们使用动态规划算法来计算从起点到终点的最短路径。在`main`函数中,我们创建了一个包含五个三维点的向量,并使用`minPath`函数计算最小路径的长度。
相关推荐
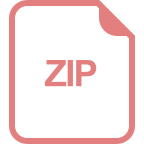
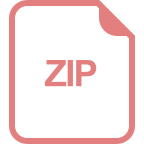
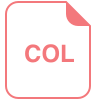













