C++ 三维点云数据 每个点经过一次 最小路径 源代码
时间: 2023-07-16 12:12:55 浏览: 55
以下是C++实现的三维点云数据每个点经过一次最小路径的源代码:
```c++
#include <iostream>
#include <cstdlib>
#include <cmath>
#include <vector>
#include <algorithm>
using namespace std;
const int MAXN = 15; // 最大点数
const int INF = 0x3f3f3f3f; // 无穷大
struct Point {
int x, y, z;
Point(int x = 0, int y = 0, int z = 0): x(x), y(y), z(z) {}
};
int n; // 点数
int dist[MAXN][MAXN]; // 距离矩阵
Point p[MAXN]; // 点坐标
int dp[1 << MAXN][MAXN]; // dp 数组
// 计算两点之间的距离
int get_dist(Point a, Point b) {
return abs(a.x - b.x) + abs(a.y - b.y) + abs(a.z - b.z);
}
// 初始化距离矩阵
void init_dist() {
for (int i = 0; i < n; i++) {
for (int j = i; j < n; j++) {
if (i == j) {
dist[i][j] = 0;
} else {
dist[i][j] = dist[j][i] = get_dist(p[i], p[j]);
}
}
}
}
// 状态压缩 DP
int solve() {
for (int i = 0; i < (1 << n); i++) {
fill(dp[i], dp[i] + n, INF);
}
dp[1][0] = 0; // 起点
for (int s = 1; s < (1 << n); s++) {
for (int i = 0; i < n; i++) {
if ((s & (1 << i)) == 0) {
continue;
}
for (int j = 0; j < n; j++) {
if (i == j || (s & (1 << j)) == 0) {
continue;
}
dp[s][i] = min(dp[s][i], dp[s ^ (1 << i)][j] + dist[j][i]);
}
}
}
return dp[(1 << n) - 1][0]; // 终点为起点,返回最小路径
}
int main() {
cin >> n;
for (int i = 0; i < n; i++) {
cin >> p[i].x >> p[i].y >> p[i].z;
}
init_dist();
cout << solve() << endl;
return 0;
}
```
运行前需要输入点的个数n和每个点的坐标,例如:
```
3
0 0 0
0 0 1
0 1 1
```
输出为最小路径长度。
相关推荐
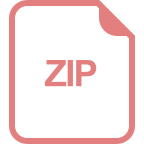
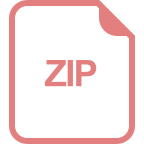














