C++三维获得点群最小路线 源代码
时间: 2023-07-15 17:12:18 浏览: 245
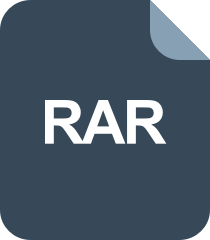
最短路径C++ 源代码
以下是一个使用 C++ 实现的三维点群最小路线算法的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <cmath>
#include <algorithm>
using namespace std;
// 定义一个点类
class Point {
public:
double x, y, z;
Point(double x, double y, double z): x(x), y(y), z(z) {}
double distance(Point p) const {
double dx = x - p.x;
double dy = y - p.y;
double dz = z - p.z;
return sqrt(dx * dx + dy * dy + dz * dz);
}
};
// 定义一个路径类
class Path {
public:
vector<Point> points;
double length() const {
double len = 0;
for (int i = 0; i < points.size() - 1; i++) {
len += points[i].distance(points[i+1]);
}
return len;
}
};
// 定义一个函数,用于计算点群的最小路线
Path findShortestPath(const vector<Point>& points) {
Path path;
path.points = points;
double minLen = path.length();
while (next_permutation(path.points.begin()+1, path.points.end())) {
double len = path.length();
if (len < minLen) {
minLen = len;
} else {
reverse(path.points.begin()+1, path.points.end());
}
}
return path;
}
int main() {
vector<Point> points = {
Point(0, 0, 0),
Point(1, 1, 1),
Point(2, 2, 2),
Point(3, 3, 3),
Point(4, 4, 4)
};
Path path = findShortestPath(points);
cout << "Shortest path length: " << path.length() << endl;
cout << "Shortest path: ";
for (auto p : path.points) {
cout << "(" << p.x << ", " << p.y << ", " << p.z << ") ";
}
cout << endl;
return 0;
}
```
该算法的思路是将点群中的所有点进行全排列,然后计算每种排列对应的路径长度,最后返回路径长度最短的那条路径。由于全排列数量为 $n!$,因此对于大规模点群,该算法的时间复杂度将非常高,不适用于实际应用。
阅读全文
相关推荐
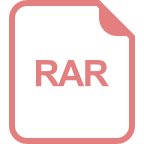








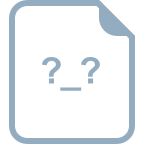
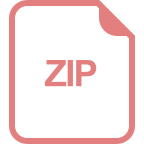
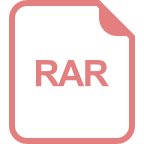
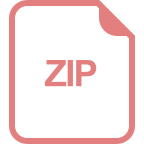
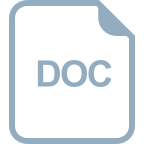
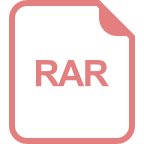
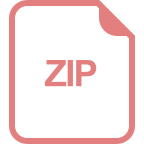
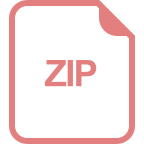