2、设计一个通用链表类,要求如下: 1)数据成员包含头指针、尾指针、链表中节点个数、顺序访问中当前节点指针和当前节点前一个节点的指针; 2)主要的成员函数包含:生成节点(在链表头、尾以及任意位置)、插入节点、删除节点、访问/修改节点、遍历链表,以及一些辅助函数等; 3)能实现两个链表的复制; 4)能实现两个链表对象的赋值; 5)在测试程序中定义两个整型链表A和B,本别插入5个元素,然后把B中的元素加入A的尾部。
时间: 2024-03-17 22:44:40 浏览: 34
好的,我可以帮你设计一个通用链表类。以下是代码实现:
```cpp
#include <iostream>
using namespace std;
// 链表节点类
template<typename T>
class Node {
public:
T data; // 数据
Node<T> *next; // 后继指针
Node<T>(T data) : data(data), next(nullptr) {} // 构造函数
};
// 链表类
template<typename T>
class LinkedList {
private:
Node<T> *head; // 头指针
Node<T> *tail; // 尾指针
int count; // 节点个数
Node<T> *current; // 当前节点指针
Node<T> *previous; // 当前节点前一个节点指针
public:
LinkedList<T>() : head(nullptr), tail(nullptr), count(0), current(nullptr), previous(nullptr) {} // 构造函数
~LinkedList<T>() { clear(); } // 析构函数
// 生成节点
void createNode(T data) {
Node<T> *node = new Node<T>(data);
if (head == nullptr) {
head = node;
tail = node;
}
else {
tail->next = node;
tail = node;
}
count++;
}
// 插入节点
void insertNode(T data, int position) {
if (position < 0 || position > count) return;
Node<T> *node = new Node<T>(data);
if (position == 0) {
node->next = head;
head = node;
}
else if (position == count) {
tail->next = node;
tail = node;
}
else {
current = head;
for (int i = 0; i < position; i++) {
previous = current;
current = current->next;
}
previous->next = node;
node->next = current;
}
count++;
}
// 删除节点
void deleteNode(int position) {
if (position < 0 || position >= count) return;
current = head;
if (position == 0) {
head = head->next;
delete current;
}
else {
for (int i = 0; i < position; i++) {
previous = current;
current = current->next;
}
previous->next = current->next;
if (current == tail) tail = previous;
delete current;
}
count--;
}
// 访问/修改节点
T& operator[](int position) {
if (position < 0 || position >= count) throw "out of range";
current = head;
for (int i = 0; i < position; i++) {
current = current->next;
}
return current->data;
}
// 遍历链表
void traverse() {
current = head;
while (current != nullptr) {
cout << current->data << " ";
current = current->next;
}
cout << endl;
}
// 清空链表
void clear() {
current = head;
while (current != nullptr) {
head = head->next;
delete current;
current = head;
}
tail = nullptr;
count = 0;
}
// 复制链表
LinkedList<T> copy() {
LinkedList<T> newList;
current = head;
for (int i = 0; i < count; i++) {
newList.createNode(current->data);
current = current->next;
}
return newList;
}
// 赋值链表
LinkedList<T>& operator=(LinkedList<T> &list) {
clear();
current = list.head;
for (int i = 0; i < list.count; i++) {
createNode(current->data);
current = current->next;
}
return *this;
}
};
int main() {
LinkedList<int> A, B;
for (int i = 1; i <= 5; i++) {
A.createNode(i);
B.createNode(i + 5);
}
A.traverse();
B.traverse();
A = A.copy();
A.traverse();
A = B;
A.traverse();
return 0;
}
```
在上述代码中,我们采用了模板类的方式,使用了节点类 `Node` 和链表类 `LinkedList`。链表类中包含了链表的基本操作,如 `createNode`、`insertNode`、`deleteNode`、`operator[]` 等等,同时也包括了链表的辅助操作,如 `traverse`、`clear`、`copy`、`operator=` 等等。
在测试程序中,我们定义了两个整型链表 `A` 和 `B`,并分别插入了 5 个元素。然后,我们将 `B` 中的元素加入到 `A` 的尾部,最后遍历输出链表 `A` 的所有元素。
相关推荐
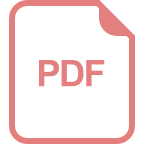
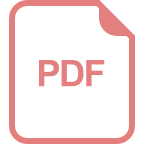














