c++求正方体、球、圆柱的表面积,抽象出一个公共的基类Container为抽象类,在其中定义一个公共的数据成员radius(此数据可以作为正方形的边长、球的半径、圆柱体底面圆半径),以及求表面积的纯虚函数area()。由此抽象类派生出要描述的三个类,利用基类指针和虚函数,分别计算三种图形的表面积。
时间: 2024-02-16 21:04:08 浏览: 88
好的,下面是一个简单的实现:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Container {
public:
Container(double r) : radius(r) {}
virtual double area() = 0;
protected:
double radius;
};
class Cube : public Container {
public:
Cube(double r) : Container(r) {}
double area() override {
return 6 * pow(radius, 2);
}
};
class Sphere : public Container {
public:
Sphere(double r) : Container(r) {}
double area() override {
return 4 * M_PI * pow(radius, 2);
}
};
class Cylinder : public Container {
public:
Cylinder(double r, double h) : Container(r), height(h) {}
double area() override {
return 2 * M_PI * radius * (radius + height);
}
private:
double height;
};
int main() {
Container* c1 = new Cube(2);
Container* c2 = new Sphere(3);
Container* c3 = new Cylinder(2, 4);
cout << "Cube surface area: " << c1->area() << endl;
cout << "Sphere surface area: " << c2->area() << endl;
cout << "Cylinder surface area: " << c3->area() << endl;
delete c1;
delete c2;
delete c3;
return 0;
}
```
在这个实现中,`Container`是一个抽象类,有一个纯虚函数`area()`,表示求表面积。`Cube`、`Sphere`、`Cylinder`都是`Container`的派生类,分别表示正方体、球和圆柱体。它们都重写了`area()`函数,根据不同的公式计算表面积。在`main()`函数中,我们通过基类指针调用了三个派生类的`area()`函数,实现了多态。
阅读全文
相关推荐
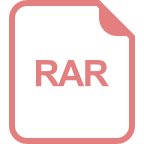
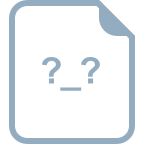

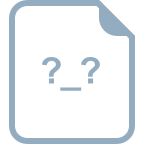
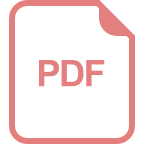
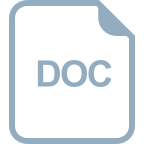
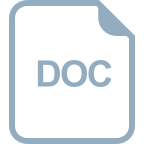






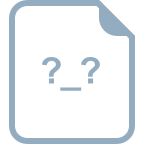
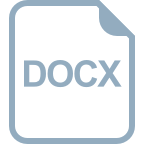