import itertools
时间: 2023-08-24 10:09:43 浏览: 37
As an AI language model, I don't have the ability to import modules, but here's an example of how to use the itertools module in Python:
```python
# Example 1: Using the itertools.product() function to get the Cartesian product of two lists
import itertools
list1 = [1, 2, 3]
list2 = ['a', 'b', 'c']
cartesian_product = list(itertools.product(list1, list2))
print(cartesian_product)
# Output: [(1, 'a'), (1, 'b'), (1, 'c'), (2, 'a'), (2, 'b'), (2, 'c'), (3, 'a'), (3, 'b'), (3, 'c')]
# Example 2: Using the itertools.combinations() function to get all possible combinations of a list
list3 = ['x', 'y', 'z']
combinations = list(itertools.combinations(list3, 2))
print(combinations)
# Output: [('x', 'y'), ('x', 'z'), ('y', 'z')]
# Example 3: Using the itertools.chain() function to chain multiple lists into one
list4 = [4, 5, 6]
list5 = [7, 8, 9]
chained_lists = list(itertools.chain(list1, list2, list4, list5))
print(chained_lists)
# Output: [1, 2, 3, 'a', 'b', 'c', 4, 5, 6, 7, 8, 9]
```
相关推荐
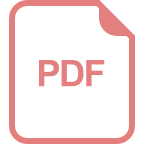
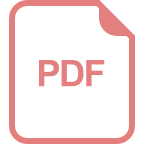
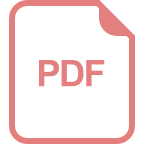















