对excel文本数据进行预处理,如去除停用词、标点符号并进行分词处理的Python代码
时间: 2024-02-05 22:04:10 浏览: 139
以下是使用Python进行文本预处理的示例代码:
```python
import jieba
import string
# 读取文本文件
with open('data.txt', 'r') as f:
text = f.read()
# 去除标点符号
text = text.translate(str.maketrans('', '', string.punctuation))
# 加载停用词表
stopwords = set()
with open('stopwords.txt', 'r') as f:
for line in f:
stopwords.add(line.strip())
# 分词并去除停用词
words = []
for word in jieba.cut(text):
if word not in stopwords:
words.append(word)
# 输出处理后的文本
print(words)
```
其中,`data.txt`是待处理的文本文件,`stopwords.txt`是停用词表文件,可以从网上下载。代码中使用了jieba分词库进行分词处理,并使用Python字符串的`translate`方法去除标点符号。最后,将去除停用词后的分词结果存储在`words`列表中,可以根据需要进行进一步的处理。
相关问题
对问答数据集进行预处理,包括文本清洗、分词、去除停用词
对问答数据集进行预处理是构建对话系统的重要步骤之一。下面是一个常见的预处理流程,包括文本清洗、分词和去除停用词。
1. 文本清洗:
- 去除HTML标签、特殊字符和非字母数字字符。
- 将文本转换为小写字母形式,以便统一处理。
- 去除多余的空格和换行符。
2. 分词:
- 使用中文分词库,如jieba分词库,对问答数据集中的句子进行分词。
- 对于英文数据集,可以使用空格或标点符号进行分词。
3. 去除停用词:
- 创建一个停用词列表,包含常见的无实际意义的词语,如“的”,“了”,“是”等。
- 对于每个问题和回答,去除其中的停用词,以减少噪音并提高关键信息的重要性。
下面是一个示例代码,使用Python和jieba分词库进行文本清洗、分词和停用词去除:
```python
import jieba
import re
def preprocess_text(text):
# 去除HTML标签和特殊字符
text = re.sub('<.*?>', '', text)
text = re.sub('[^\w\s]', '', text)
# 转换为小写字母形式
text = text.lower()
# 去除多余的空格和换行符
text = re.sub('\s+', ' ', text)
text = text.strip()
return text
def tokenize_text(text):
# 使用jieba分词进行分词
tokens = jieba.lcut(text)
return tokens
def remove_stopwords(tokens, stopwords):
# 去除停用词
filtered_tokens = [token for token in tokens if token not in stopwords]
return filtered_tokens
# 读取停用词列表
with open('stopwords.txt', 'r', encoding='utf-8') as f:
stopwords = [line.strip() for line in f]
# 示例文本
text = "这是一个示例文本,包含一些特殊字符和停用词。"
# 文本预处理
cleaned_text = preprocess_text(text)
# 分词
tokens = tokenize_text(cleaned_text)
# 去除停用词
filtered_tokens = remove_stopwords(tokens, stopwords)
print(filtered_tokens)
```
请注意,以上代码只是一个简单的示例,实际的预处理过程可能需要根据具体需求进行更详细的处理。同时,在使用jieba分词库时,您可能需要根据具体情况进行自定义分词字典的加载和设置。希望这个示例能对您有所帮助!
用pycharm编写新闻分类,根据新闻文本中的内容,进行文本预处理,建模等操作,从而可以自动将新闻划分到最可能的类别中,节省人力资源。 具体实现内容包括: ◆能够对文本数据进行预处理。【文本清洗, 分词,去除停用词,文本向量化等操作。】 ◆能够通过Python统计词频,生成词云图。【描述性统计分析】 ◆能够通过方差分析,进行特征选择。【验证性统计分析】 ◆能够根据文本内容,对文本数据进行分类。【统计建模】
首先,需要准备新闻文本数据集,并进行文本预处理。文本预处理过程包括以下步骤:
1. 去除停用词:将一些常用的词汇(如“的”、“是”、“在”等)从文本中去除,因为这些词汇对分类结果没有贡献。
2. 分词:将文本分割成一个个词汇。可以使用中文分词工具,如jieba。
3. 文本清洗:去除文本中的特殊字符、数字、标点符号等。
4. 文本向量化:将文本转换成数值表示,可以使用词袋模型、TF-IDF等方法。
下面是一个简单的示例代码:
```python
import jieba
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.model_selection import train_test_split
import pandas as pd
# 读取新闻数据集
data = pd.read_csv('news.csv')
# 分词
data['content'] = data['content'].apply(lambda x: ' '.join(jieba.cut(x)))
# 去除停用词
stop_words = set(open('stopwords.txt', encoding='utf-8').read().splitlines())
data['content'] = data['content'].apply(lambda x: ' '.join([word for word in x.split() if word not in stop_words]))
# 文本向量化
vectorizer = TfidfVectorizer(max_features=5000)
X = vectorizer.fit_transform(data['content'])
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, data['label'], test_size=0.2, random_state=42)
```
接下来,可以使用Python统计词频,生成词云图。词云图可以帮助我们直观地了解文本中的关键词汇。
```python
import matplotlib.pyplot as plt
from wordcloud import WordCloud
# 统计词频
word_freq = dict(zip(vectorizer.get_feature_names(), X.sum(axis=0).tolist()[0]))
sorted_word_freq = sorted(word_freq.items(), key=lambda x: x[1], reverse=True)
# 生成词云图
wordcloud = WordCloud(width=800, height=800, background_color='white', max_words=100).generate_from_frequencies(word_freq)
plt.figure(figsize=(8, 8), facecolor=None)
plt.imshow(wordcloud)
plt.axis("off")
plt.tight_layout(pad=0)
plt.show()
```
方差分析可以用来进行特征选择,即选择对分类结果有较大贡献的特征。可以使用ANOVA(方差分析)或卡方检验等方法进行特征选择。
最后,可以使用机器学习算法对文本进行分类。常用的分类算法包括朴素贝叶斯、支持向量机、随机森林等。以朴素贝叶斯为例:
```python
from sklearn.naive_bayes import MultinomialNB
from sklearn.metrics import classification_report
# 训练朴素贝叶斯模型
clf = MultinomialNB()
clf.fit(X_train, y_train)
# 在测试集上评估模型性能
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
```
阅读全文
相关推荐
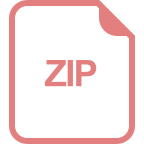
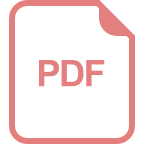
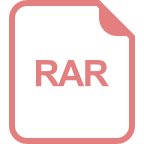
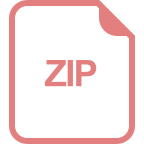
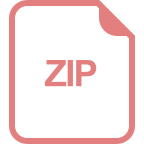
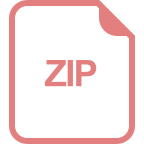
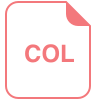
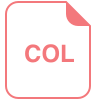







