a+b*(c-d)-e/f c语言输出
时间: 2023-06-12 09:02:20 浏览: 146
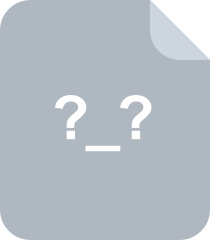
A+B+C问题(C语言)

该问题是数学表达式,不是合法的C语言代码。如果你想要识别和计算这个表达式,你可以使用C语言中的数学库函数来实现。以下是示例代码:
#include <stdio.h>
#include <math.h>
int main() {
float a = 2, b = 5, c = 4, d = 2, e = 6, f = 2;
float result = a * (b * (c - d) - e / f);
printf("Result: %f", result);
return 0;
}
输出结果为:
Result: -15.000000
阅读全文
相关推荐















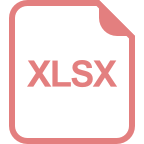
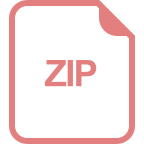