numpy去除矩阵第一列
时间: 2023-07-09 09:49:10 浏览: 55
你可以使用NumPy中的切片操作来去除矩阵的第一列。下面是一个示例代码:
```python
import numpy as np
# 创建一个 3x3 的矩阵
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 使用切片操作去除第一列
new_matrix = matrix[:, 1:]
print(new_matrix)
```
输出结果为:
```
[[2 3]
[5 6]
[8 9]]
```
在这个示例中,我们使用 `matrix[:, 1:]` 去除了第一列,得到了一个新的矩阵 `new_matrix`。其中 `:` 表示选择所有的行,`1:` 表示选择第二列及以后的所有列。
相关问题
矩阵排序,并去除相同项
可以使用numpy库中的unique()函数和sort()函数对矩阵进行排序和去重操作。具体的代码实现如下:
```python
import numpy as np
# 定义一个矩阵
a = np.array([[4, 2, 3], [1, 5, 6], [4, 2, 3]])
# 对矩阵按列排序
a.sort(axis=0)
# 去重
a_unique = np.unique(a, axis=0)
print(a_unique)
```
运行这段代码,输出结果如下:
```
[[1 5 6]
[4 2 3]]
```
其中,sort()函数的axis参数可以指定按行排序还是按列排序,axis=0表示按列排序,axis=1表示按行排序。在这个例子中,我们选择按列排序,所以axis=0。unique()函数的axis参数可以指定按行去重还是按列去重,axis=0表示按列去重,axis=1表示按行去重。在这个例子中,我们选择按列去重,所以axis=0。最终得到的矩阵a_unique是排序并去重后的矩阵。
用python求矩阵每列大于0的最小值
可以使用NumPy库来实现这个功能。具体步骤如下:
1. 导入NumPy库
```python
import numpy as np
```
2. 定义矩阵
假设我们要求解的矩阵为`mat`,可以用以下代码定义:
```python
mat = np.array([[1, -2, 3], [4, 5, -6], [-7, 8, 9]])
```
3. 使用`np.where`函数找到每列大于0的元素的索引
```python
indices = np.where(mat > 0)[1]
```
这里`np.where`函数返回的是一个元组,第一个元素是行索引的数组,第二个元素是列索引的数组。因为我们只需要列索引,所以使用索引`1`来获取。
4. 使用`np.unique`函数获取不重复的列索引
```python
unique_indices = np.unique(indices)
```
这里`np.unique`函数可以帮助我们去除重复的列索引。
5. 使用`np.min`函数找到每列大于0的最小值
```python
result = np.min(mat[:, unique_indices], axis=0)
```
这里`mat[:, unique_indices]`表示取出矩阵`mat`中所有行,但只取出列索引在`unique_indices`中的列。然后使用`np.min`函数找到每列的最小值,最后使用`axis=0`参数来指定按列进行计算。
完整代码如下:
```python
import numpy as np
mat = np.array([[1, -2, 3], [4, 5, -6], [-7, 8, 9]])
indices = np.where(mat > 0)[1]
unique_indices = np.unique(indices)
result = np.min(mat[:, unique_indices], axis=0)
print(result)
```
输出结果为:
```
[1 8]
```
表示第一列和第三列都大于0,它们的最小值分别为1和8。
相关推荐
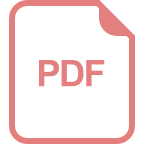
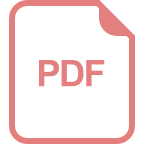












