用python实现巴特沃斯滤波器
时间: 2023-05-22 19:04:25 浏览: 68
可以的,以下是一个巴特沃斯滤波器的 Python 实现示例:
```python
from scipy.signal import butter, filtfilt
import numpy as np
def butter_bandpass(lowcut, highcut, fs, order=5):
nyq = 0.5 * fs
low = lowcut / nyq
high = highcut / nyq
b, a = butter(order, [low, high], btype='band')
return b, a
def butter_bandpass_filter(data, lowcut, highcut, fs, order=5):
b, a = butter_bandpass(lowcut, highcut, fs, order=order)
y = filtfilt(b, a, data)
return y
# 示例
# 生成随机信号
fs = 100.0
t = np.arange(0, 1, 1/fs)
noise = np.random.randn(len(t))
signal = np.sin(2*np.pi*10*t) + np.sin(2*np.pi*30*t) + noise
# 设计巴特沃斯滤波器,滤除 20 Hz - 25 Hz 以外的信号
lowcut = 20.0
highcut = 25.0
order = 3
y = butter_bandpass_filter(signal, lowcut, highcut, fs, order=order)
```
这里用到了 SciPy 库中的 `butter` 和 `filtfilt` 函数,分别用于设计巴特沃斯滤波器和对信号进行滤波。函数 `butter_bandpass` 设计了一个带通滤波器(bandpass filter),函数 `butter_bandpass_filter` 对输入的数据进行滤波,并返回滤波后的结果。在示例中,我们生成了一个包含两个正弦波和噪声的信号,然后将它的 20 Hz - 25 Hz 之外的部分滤除,得到了滤波后的信号。
相关推荐
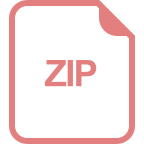














