python的多线程之间参数传递,共享变量,简单代码示例?
时间: 2023-10-20 14:21:02 浏览: 132
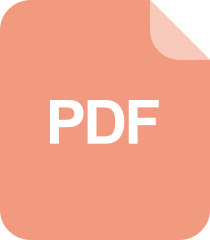
python 多线程共享全局变量的优劣

可以的,对于Python的多线程之间参数传递和共享变量,可以使用Lock和Queue来实现。以下是一个简单的代码示例:
```python
import threading
import time
from queue import Queue
# 定义全局变量
num = 0
# 定义线程锁
lock = threading.Lock()
# 定义队列
queue = Queue()
# 定义线程函数,将num加一
def add_num():
global num
# 加锁
lock.acquire()
num += 1
# 释放锁
lock.release()
time.sleep(1)
# 定义线程函数,将num乘二
def mul_num():
global num
# 加锁
lock.acquire()
num *= 2
# 释放锁
lock.release()
time.sleep(1)
# 定义线程函数,将num加一后放入队列
def add_num_to_queue():
add_num()
queue.put(num)
# 定义线程函数,将队列中的数值乘二
def mul_num_from_queue():
num = queue.get()
num *= 2
queue.put(num)
# 创建线程
thread1 = threading.Thread(target=add_num_to_queue)
thread2 = threading.Thread(target=mul_num_from_queue)
# 启动线程
thread1.start()
thread2.start()
# 等待线程结束
thread1.join()
thread2.join()
# 输出结果
print("最终结果:", queue.get())
```
在代码中,我们使用了Lock来保证了全局变量num的操作互斥进行,使用Queue来实现了线程之间的变量传递。
阅读全文
相关推荐
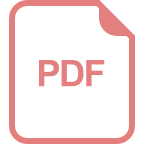
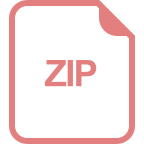















