从数据加载到去除停用词和提取文本征向量前的 python 代码。代码要清 晰易读,要有适当的注释。
时间: 2024-03-01 10:49:44 浏览: 22
好的,下面是一个从数据加载到去除停用词和提取文本特征向量的示例代码:
```python
import pandas as pd
import numpy as np
import re
import jieba
from gensim.models import Word2Vec
# Step 1: 加载数据
def load_data(file_path):
data = pd.read_csv(file_path)
return data
# Step 2: 数据预处理
def clean_text(text):
# 去除标点符号
text = re.sub(r'[^\w\s]','',text)
# 分词
seg_list = jieba.cut(text)
# 去停用词
stopwords = open("stopwords.txt", "r", encoding="utf-8").read().split("\n")
words = [word for word in seg_list if word not in stopwords]
return words
# Step 3: 构建词表和词向量
def build_word2vec_model(sentences):
model = Word2Vec(sentences, size=100, window=5, min_count=5, workers=4)
model.save("word2vec.model")
return model
# Step 4: 文本编码
def encode_text(text, vocab):
return [vocab[word] for word in text if word in vocab]
# Step 5: 提取文本特征向量
def extract_features(data):
word2vec_model = Word2Vec.load("word2vec.model")
vocab = word2vec_model.wv.vocab
features = np.zeros((len(data), 100))
for i in range(len(data)):
text = data.iloc[i]["text"]
words = clean_text(text)
sentence = encode_text(words, vocab)
for word_index in sentence:
features[i] += word2vec_model.wv[word_index]
features[i] /= len(sentence)
return features
# 示例代码的使用
data = load_data("data.csv")
sentences = data["text"].apply(clean_text).tolist()
word2vec_model = build_word2vec_model(sentences)
features = extract_features(data)
```
在这段代码中,首先通过 `load_data` 函数加载数据。接着,使用 `clean_text` 函数进行数据预处理,包括去除标点符号、分词和去除停用词。然后,使用 `build_word2vec_model` 函数构建词表和词向量模型。接着,使用 `encode_text` 函数将文本编码成数字序列。最后,使用 `extract_features` 函数提取文本特征向量。需要注意的是,这里的 `vocab` 是从词向量模型中获取的。
相关推荐
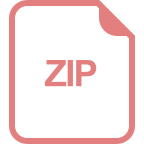
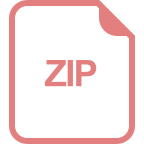
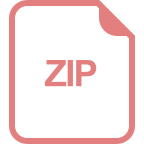














