unity点击button使物体向右旋转
时间: 2023-09-25 08:11:25 浏览: 92
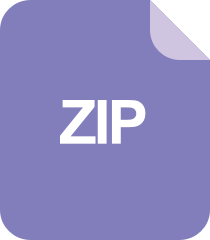
点击Button,对button进行旋转
可以通过以下步骤实现在Unity中点击按钮使物体向右旋转:
1. 创建一个3D物体,例如一个立方体。
2. 在场景中创建一个UI Button。
3. 在Button上添加一个OnClick事件。
4. 将需要旋转的物体作为目标拖到OnClick事件的目标位置。
5. 在OnClick事件中添加一个C#脚本,例如以下示例代码:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class RotateObject : MonoBehaviour
{
public float speed = 10f; // 旋转速度
void Update()
{
if (Input.GetMouseButton(0))
{
// 当鼠标左键按下时旋转物体
transform.Rotate(Vector3.right * speed * Time.deltaTime);
}
}
}
```
6. 将RotateObject脚本添加到需要旋转的物体上。
7. 现在,当点击按钮时,物体将向右旋转。
阅读全文
相关推荐
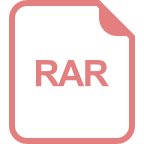
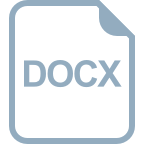
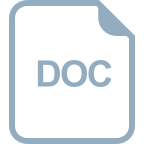
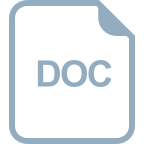
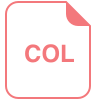

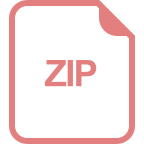
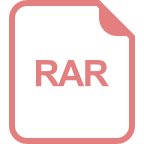
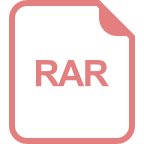
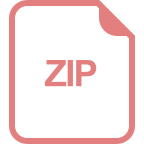
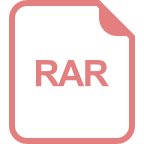
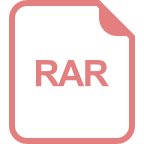