while (getline(infile, str[l])) { cout << str[l++] << endl; }
时间: 2024-04-27 19:20:56 浏览: 55
这段代码使用了 `std::getline()` 函数从 `infile` 流中读取每一行文本,并将其存储到 `str` 数组中。每次读取成功后,会使用 `cout` 打印出该行文本,并通过 `l++` 将 `l` 值自增,以便在下一次读取时将文本存储到下一个数组元素中。
需要注意的是,这段代码没有对 `str` 数组的大小进行检查,如果文件中包含的行数超过了 `str` 数组的大小,就会发生数组越界错误。因此,在使用这段代码时,需要确保 `str` 数组的大小足够存储文件中的所有行。
相关问题
void loginMenu() { cout << "请选择登录类型:" << endl; cout << "1. 学生登录" << endl; cout << "2. 管理员登录" << endl; cout << "3. 注册" << endl; cout << "0. 退出" << endl; 在不使用数据库的前提下,怎么将注册得到的信息用于登录、
如果不使用数据库,可以将注册的用户信息保存到文件中,例如一个文本文件。在注册时,将用户输入的信息写入到文件中,每个用户的信息占用一行,不同字段之间可以使用逗号、空格或其他分隔符进行分隔。在登录时,读取保存用户信息的文件,根据用户输入的用户名或邮箱查找对应的用户信息,然后进行密码校验,如果校验通过,则允许用户登录。
下面是一个简单的示例,演示如何将用户信息保存到文件中,并在登录时使用文件中的信息进行校验。
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
// 定义用户信息结构体
struct UserInfo {
string username;
string password;
string email;
};
// 注册函数,将用户信息保存到文件中
void registerUser() {
UserInfo user;
cout << "请输入用户名:";
cin >> user.username;
cout << "请输入密码:";
cin >> user.password;
cout << "请输入邮箱:";
cin >> user.email;
// 将用户信息写入到文件中
ofstream outfile("user.txt", ios::app);
outfile << user.username << " " << user.password << " " << user.email << endl;
outfile.close();
cout << "注册成功!" << endl;
}
// 登录函数,从文件中读取用户信息进行校验
void loginUser() {
string username, password;
cout << "请输入用户名或邮箱:";
cin >> username;
cout << "请输入密码:";
cin >> password;
// 从文件中读取用户信息进行校验
ifstream infile("user.txt");
string line;
bool found = false;
while (getline(infile, line)) {
size_t pos = line.find(" ");
string name = line.substr(0, pos);
string rest = line.substr(pos + 1);
pos = rest.find(" ");
string pwd = rest.substr(0, pos);
string email = rest.substr(pos + 1);
if (name == username || email == username) {
found = true;
if (pwd == password) {
cout << "登录成功!" << endl;
} else {
cout << "密码错误!" << endl;
}
break;
}
}
infile.close();
if (!found) {
cout << "用户名或邮箱不存在!" << endl;
}
}
// 登录菜单函数
void loginMenu() {
int choice;
do {
cout << "请选择登录类型:" << endl;
cout << "1. 学生登录" << endl;
cout << "2. 管理员登录" << endl;
cout << "3. 注册" << endl;
cout << "0. 退出" << endl;
cin >> choice;
switch (choice) {
case 1:
cout << "学生登录" << endl;
loginUser();
break;
case 2:
cout << "管理员登录" << endl;
loginUser();
break;
case 3:
cout << "注册" << endl;
registerUser();
break;
case 0:
cout << "退出" << endl;
break;
default:
cout << "无效选择,请重新输入!" << endl;
break;
}
} while (choice != 0);
}
int main() {
loginMenu();
return 0;
}
```
#include<iostream> #include<fstream> using namespace std; int main() { char ch; int i; float f; char str1[10], str2[10]; ifstream in("d:\test"); if (!in) { cout << "cannot open file."; return 0; } in >> i >> f >> ch >> str1 >> str2; cout << i << "" << f << "" << ch << "\n"; cout << str1 << str2 << endl; in.close(); }
根据您提供的代码,以下是一些可能的改进建议:
1. 文件路径中的反斜杠应该使用双反斜杠:由于反斜杠是C++中的转义字符,因此在文件路径中使用时需要使用两个反斜杠。因此,将`ifstream in("d:\test");`改为`ifstream in("d:\\test");`。
2. 检查文件是否成功读取:在读取文件之后,您应该检查文件是否成功读取,以确保读取的数据是有效的。例如,您可以使用以下代码检查读取的整数是否在有效范围内:
```
if (i < 0 || i > 100) {
cout << "Invalid data: i=" << i << endl;
return 0;
}
```
3. 使用更好的变量名:使用更好的变量名可以使代码更易于理解和维护。例如,`ch`可以改为`character`,`i`可以改为`integerData`,等等。
4. 检查文件是否成功关闭:在使用完文件之后,您应该检查文件是否成功关闭。例如,您可以使用以下代码:
```
in.close();
if (in.is_open()) {
cout << "Error: failed to close file." << endl;
return 0;
}
```
5. 使用更安全的函数:在读取字符串时,使用`cin`和`cout`等函数可能会导致缓冲区溢出和其他安全问题。在C++11中,您可以使用更安全的`std::getline`函数来读取字符串。例如,您可以使用以下代码:
```
std::getline(in, str1, '\n');
std::getline(in, str2, '\n');
```
6. 错误处理:需要处理读取数据时的错误,比如输入的数据类型不匹配或者读取的数据格式不正确。
下面是改进后的代码示例:
```
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
char character;
int integerData;
float floatData;
string str1, str2;
ifstream in("d:\\test");
if (!in.is_open()) {
cout << "Cannot open file." << endl;
return 0;
}
if (!(in >> integerData >> floatData >> character >> str1 >> str2)) {
cout << "Error reading data." << endl;
return 0;
}
if (integerData < 0 || integerData > 100) {
cout << "Invalid data: integerData=" << integerData << endl;
return 0;
}
cout << integerData << " " << floatData << " " << character << endl;
cout << str1 << " " << str2 << endl;
in.close();
if (in.is_open()) {
cout << "Error: failed to close file." << endl;
return 0;
}
return 0;
}
```
请注意,这只是改进的示例,具体的改进取决于您的具体需求和代码的实际情况。
阅读全文
相关推荐
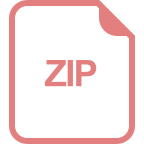
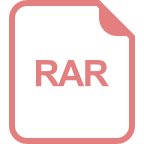
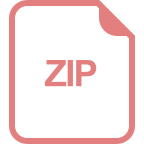












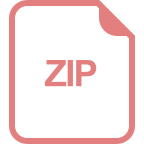
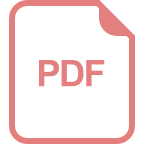