python遍历文件夹中所有txt文件。取第一个txt文件名的后六个字符,与后六个txt文件名的后六个字符比较,如果相同的有六个,则print这六个txt,如果相同的不足六个,则舍弃前面几个相同的txt文件,从不同的txt文件继续此操作
时间: 2024-02-26 22:57:29 浏览: 61
好的,下面是 Python 的代码实现:
```python
import os
# 遍历文件夹,获取所有txt文件的绝对路径
def get_txt_files(path):
txt_files = []
for root, dirs, files in os.walk(path):
for file in files:
if file.endswith('.txt'):
txt_files.append(os.path.join(root, file))
return txt_files
# 获取文件名的后六个字符
def get_last_six_chars(file):
return file[-10:-4]
# 比较文件名后六个字符,输出相同的文件
def compare_files(files):
last_six_chars = get_last_six_chars(files[0])
same_files = [files[0]]
for file in files[1:]:
if get_last_six_chars(file) == last_six_chars:
same_files.append(file)
else:
if len(same_files) >= 6:
print(same_files)
same_files = [file]
last_six_chars = get_last_six_chars(file)
if len(same_files) >= 6:
print(same_files)
# 主函数
if __name__ == "__main__":
path = 'your_folder_path' # 文件夹路径
txt_files = get_txt_files(path)
txt_files.sort(key=os.path.getmtime) # 按时间排序
compare_files(txt_files)
```
需要注意的是,这段代码假设每个 txt 文件名都至少有 10 个字符。如果文件名长度不足 10 个字符,会导致程序出错。
阅读全文
相关推荐







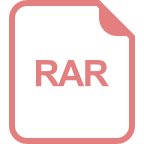







