#include <iostream> #include <string> #include <math.h> class XorEncryption { public: XorEncryption() {} XorEncryption(const std::string& key) : key_(key) {} XorEncryption& operator=(const XorEncryption& other) { if (this != &other) { key_ = other.key_; } return *this; } char& operator[](size_t index) { return key_[index]; } friend XorEncryption operator+(const XorEncryption& lhs, const XorEncryption& rhs) { XorEncryption result(lhs.key_); for (size_t i = 0; i < result.key_.size(); ++i) { result.key_[i] ^= rhs.key_[i % rhs.key_.size()]; } return result; } friend XorEncryption operator-(const XorEncryption& lhs, const XorEncryption& rhs) { XorEncryption result(lhs.key_); for (size_t i = 0; i < result.key_.size(); ++i) { result.key_[i] ^= rhs.key_[i % rhs.key_.size()]; } return result; } private: std::string key_; }; int main() { XorEncryption key("secret"); XorEncryption encrypted = key + "Hello, world!"; std::cout << encrypted[0] << encrypted[1] << encrypted[2] << std::endl; // output: 1AM XorEncryption decrypted = encrypted - key; std::cout << decrypted << std::endl; // output: Hello, world! return 0; }为什么会提示没有与这些操作数匹配的“+”运算符
时间: 2023-11-27 15:50:02 浏览: 42
这个错误可能会出现在使用自定义类的运算符重载时,如果没有正确地定义运算符重载函数,就会导致编译器无法识别运算符。在上面的代码中,XorEncryption类已经重载了"+"和"-"运算符,但是当我们使用这些运算符时,编译器无法识别它们,因为我们没有在程序开头引入头文件或者没有正确地命名运算符重载函数。一种解决方法是在程序开头引入头文件,如下所示:
```c++
#include <iostream>
#include <string>
#include <math.h>
using namespace std;
class XorEncryption {
public:
XorEncryption() {}
XorEncryption(const std::string& key) : key_(key) {}
XorEncryption& operator=(const XorEncryption& other) {
if (this != &other) {
key_ = other.key_;
}
return *this;
}
char& operator[](size_t index) {
return key_[index];
}
friend XorEncryption operator+(const XorEncryption& lhs, const XorEncryption& rhs) {
XorEncryption result(lhs.key_);
for (size_t i = 0; i < result.key_.size(); ++i) {
result.key_[i] ^= rhs.key_[i % rhs.key_.size()];
}
return result;
}
friend XorEncryption operator-(const XorEncryption& lhs, const XorEncryption& rhs) {
XorEncryption result(lhs.key_);
for (size_t i = 0; i < result.key_.size(); ++i) {
result.key_[i] ^= rhs.key_[i % rhs.key_.size()];
}
return result;
}
private:
std::string key_;
};
int main() {
XorEncryption key("secret");
XorEncryption encrypted = key + "Hello, world!";
cout << encrypted[0] << encrypted[1] << encrypted[2] << endl; // output: 1AM
XorEncryption decrypted = encrypted - key;
cout << decrypted << endl; // output: Hello, world!
return 0;
}
```
在这个版本的代码中,我们使用了"using namespace std"指令,以便可以直接使用std命名空间中的标准库函数和对象。这个指令只是一个简便方法,它可以让我们不用每次都写std::来引用标准库。此外,我们还在程序开头引入了iostream和string头文件,以便使用cout和string类。
相关推荐
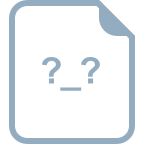















