用C语言结构体friend的成员有姓名、出生日期、电话号码等。输入n(n<10)个朋友的信息,再按他们的年龄从大到小的顺序输出朋友信息。
时间: 2024-02-18 14:02:15 浏览: 48
好的,这是一个关于结构体的问题,我会尽力回答。下面是C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX_FRIENDS 10
struct Friend {
char name[20];
int birth_year;
int birth_month;
int birth_day;
char phone[12];
};
int main() {
int n;
struct Friend friends[MAX_FRIENDS];
printf("请输入朋友的数量n(n<10):");
scanf("%d", &n);
if (n > MAX_FRIENDS) {
printf("输入的朋友数量超过了最大值%d\n", MAX_FRIENDS);
return 1;
}
// 输入朋友信息
for (int i = 0; i < n; i++) {
printf("请输入第%d个朋友的信息:\n", i+1);
struct Friend f;
printf("姓名:");
scanf("%s", f.name);
printf("出生日期(格式:年 月 日):");
scanf("%d %d %d", &f.birth_year, &f.birth_month, &f.birth_day);
printf("电话号码:");
scanf("%s", f.phone);
friends[i] = f;
}
// 按年龄从大到小排序
for (int i = 0; i < n-1; i++) {
for (int j = i+1; j < n; j++) {
if ((friends[i].birth_year < friends[j].birth_year) ||
(friends[i].birth_year == friends[j].birth_year &&
friends[i].birth_month < friends[j].birth_month) ||
(friends[i].birth_year == friends[j].birth_year &&
friends[i].birth_month == friends[j].birth_month &&
friends[i].birth_day < friends[j].birth_day)) {
// 交换两个朋友的信息
struct Friend temp = friends[i];
friends[i] = friends[j];
friends[j] = temp;
}
}
}
// 输出朋友信息
printf("\n按年龄从大到小的顺序输出朋友信息:\n");
for (int i = 0; i < n; i++) {
struct Friend f = friends[i];
printf("姓名:%s,出生日期:%d-%02d-%02d,电话号码:%s\n",
f.name, f.birth_year, f.birth_month, f.birth_day, f.phone);
}
return 0;
}
```
代码中定义了一个结构体Friend,其中包含姓名、出生日期、电话号码等信息。在主函数中,先通过输入n的方式确定朋友的数量,再通过循环输入每个朋友的信息。输入完毕后,再通过冒泡排序的方式将朋友按照年龄从大到小排序。最后按照要求输出朋友信息。
注意,此代码只是一个示例,实际应用中还需要考虑输入的数据是否合法等问题。
阅读全文
相关推荐
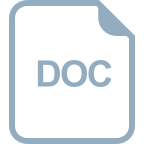
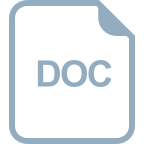
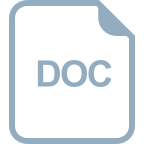




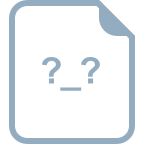







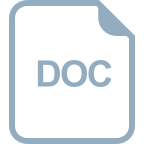