python提取label=1列的文本内容
时间: 2023-12-02 15:06:24 浏览: 34
假设你有一个名为data的DataFrame,其中包含一个名为label的列,你可以使用以下代码提取label=1列的文本内容:
```python
text_data = data.loc[data['label'] == 1, 'text_column_name'].tolist()
```
其中,`text_column_name`是你想要提取文本内容的列的名称。这将返回一个包含所有符合条件的文本内容的列表。
相关问题
Python文本词频统计提取地名
在Python中,文本词频统计是一个常见的自然语言处理任务,特别是在处理文本挖掘和信息检索时。对于地名的提取,通常涉及到命名实体识别(NER),特别是地点(Location)类型的实体。Python有一些库可以方便地完成这个任务,比如`nltk`、`spaCy`、`geopy`等。
例如,`nltk`中的`FreqDist`可以用来统计单词频率,`Pattern`库或`TextBlob`提供了一些预训练的命名实体识别模型。`spaCy`是一个强大的库,它有内置的实体识别功能,包括地点名称。
以下是一个简单的例子,使用`nltk`和`spaCy`提取文本中的地名:
```python
import nltk
from spacy import load
# 使用nltk进行词频统计
text = "北京是中国的首都,而纽约是美国的城市。"
tokens = nltk.word_tokenize(text)
freq_dist = nltk.FreqDist(tokens)
for word, freq in freq_dist.items():
if word.lower() in ['北京', '纽约']: # 假设我们只关心这两个特定地名
print(f"{word}: {freq}")
# 使用spaCy提取地点
nlp = load('en_core_web_sm') # 英语模型,根据需要选择其他语言模型
doc = nlp(text)
for ent in doc.ents:
if ent.label_ == 'GPE': # GPE代表地理位置
print(f"{ent.text}: {ent.label_}")
python文本中提取名词
为了在Python中提取文本中的名词,我们可以使用自然语言处理(NLP)库NLTK(Natural Language Toolkit)。引用中的代码展示了一个名词短语提取器的实现。该提取器通过查找词性标注后的文本中的名词单词,并向前、向后扩展,直到遇到非名词单词为止。具体的代码如下:
```python
import nltk
def extract_np(tagged_sent):
grammar = r'NP: {<DT>?<JJ>*<NN>}' # 定义名词短语的语法规则
cp = nltk.RegexpParser(grammar)
result = cp.parse(tagged_sent)
return result
nps = []
tagged_tokens = nltk.pos_tag(tokens) # 对文本进行词性标注
for tagged_sent in tagged_tokens:
tree = extract_np(tagged_sent) # 提取名词短语
for subtree in tree.subtrees():
if subtree.label() == 'NP':
t = subtree
t = ' '.join(word for word, tag in t.leaves()) # 将名词短语拼接成字符串
nps.append(t)
```
以上代码首先使用`nltk.pos_tag()`函数对文本进行词性标注,然后将标注结果传递给`extract_np()`函数进行名词短语提取。最后,将提取得到的名词短语存储在列表`nps`中。请注意,这只是提取名词的一种方法,还可以根据实际需求进行调整和改进。引用和提供了关于NLP和名词短语提取的更详细的信息。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [chatgpt赋能python:Python提取名词:利用NLP技术进行文本分析的高效方法](https://blog.csdn.net/laingziwei/article/details/131319121)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [Python实现基于自然语言处理工具NLTK的名词短语提取器](https://blog.csdn.net/Jack_user/article/details/130976216)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
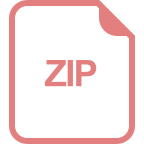
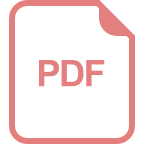












