用C++实现车辆管理系统代码
时间: 2024-03-21 22:44:27 浏览: 53
请注意,由于车辆管理系统的实现涉及到多个方面,代码实现可能与具体需求有关,因此下面的代码仅供参考,不一定适用于所有情况。同时,车辆管理系统的实现还需要涉及到界面设计、数据存储、异常处理等方面,因此下面的代码只是实现车辆信息的基本操作。
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义结构体保存车辆信息
struct Car {
string name; // 车名
string brand; // 品牌
string model; // 型号
int price; // 价格
};
// 定义函数实现车辆信息的录入
void addCar(Car cars[], int &count) {
cout << "请输入车名:";
cin >> cars[count].name;
cout << "请输入品牌:";
cin >> cars[count].brand;
cout << "请输入型号:";
cin >> cars[count].model;
cout << "请输入价格:";
cin >> cars[count].price;
count++; // 数量加1
}
// 定义函数实现车辆信息的查询
void searchCar(Car cars[], int count) {
cout << "请输入要查询的车名:";
string name;
cin >> name;
bool found = false; // 是否找到标记
for (int i = 0; i < count; i++) {
if (cars[i].name == name) {
cout << "车名:" << cars[i].name << endl;
cout << "品牌:" << cars[i].brand << endl;
cout << "型号:" << cars[i].model << endl;
cout << "价格:" << cars[i].price << endl;
found = true;
break;
}
}
if (!found) {
cout << "未找到车辆信息!" << endl;
}
}
// 定义函数实现车辆信息的修改
void modifyCar(Car cars[], int count) {
cout << "请输入要修改的车名:";
string name;
cin >> name;
bool found = false; // 是否找到标记
for (int i = 0; i < count; i++) {
if (cars[i].name == name) {
cout << "请输入新的品牌:";
cin >> cars[i].brand;
cout << "请输入新的型号:";
cin >> cars[i].model;
cout << "请输入新的价格:";
cin >> cars[i].price;
found = true;
break;
}
}
if (!found) {
cout << "未找到车辆信息!" << endl;
} else {
cout << "车辆信息修改成功!" << endl;
}
}
// 定义函数实现车辆信息的删除
void deleteCar(Car cars[], int &count) {
cout << "请输入要删除的车名:";
string name;
cin >> name;
bool found = false; // 是否找到标记
for (int i = 0; i < count; i++) {
if (cars[i].name == name) {
for (int j = i; j < count - 1; j++) {
cars[j] = cars[j + 1]; // 后续车辆信息向前移动
}
count--; // 数量减1
found = true;
break;
}
}
if (!found) {
cout << "未找到车辆信息!" << endl;
} else {
cout << "车辆信息删除成功!" << endl;
}
}
int main() {
Car cars[100]; // 最多保存100辆车辆信息
int count = 0; // 当前保存的车辆数量
while (true) {
cout << "请选择操作:1.录入 2.查询 3.修改 4.删除 5.退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
addCar(cars, count);
break;
case 2:
searchCar(cars, count);
break;
case 3:
modifyCar(cars, count);
break;
case 4:
deleteCar(cars, count);
break;
case 5:
cout << "谢谢使用!" << endl;
return 0;
default:
cout << "请输入正确的操作序号!" << endl;
break;
}
}
return 0;
}
```
阅读全文
相关推荐
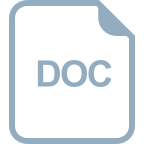
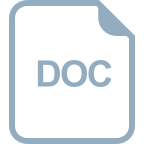
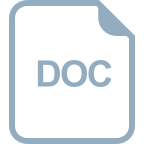
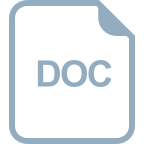
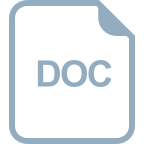

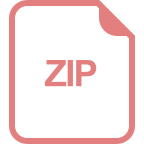
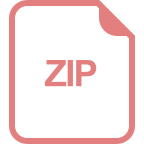
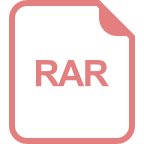
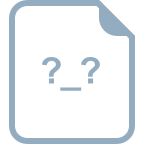
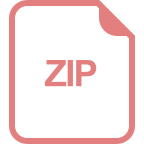
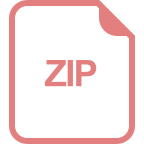
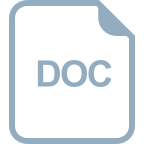
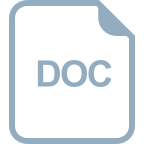
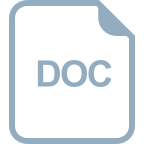
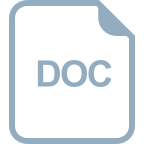
