用C++写车辆管理系统代码
时间: 2023-11-08 09:05:45 浏览: 162
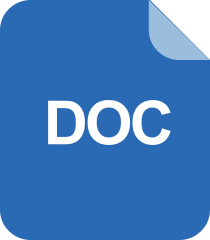
C++程序设计车辆管理系统实验报告附源代码.doc

以下是一个简单的基于控制台的车辆管理系统的C++代码,包括添加车辆、查看车辆信息、修改车辆信息和删除车辆等功能:
```cpp
#include <iostream>
#include <vector>
#include <string>
using namespace std;
struct Car {
string brand;
string model;
int year;
string color;
string licensePlate;
};
vector<Car> carList;
void addCar() {
Car newCar;
cout << "请输入品牌:";
cin >> newCar.brand;
cout << "请输入型号:";
cin >> newCar.model;
cout << "请输入生产年份:";
cin >> newCar.year;
cout << "请输入颜色:";
cin >> newCar.color;
cout << "请输入车牌号码:";
cin >> newCar.licensePlate;
carList.push_back(newCar);
cout << "车辆信息已添加!" << endl;
}
void viewCar() {
if (carList.empty()) {
cout << "没有车辆信息!" << endl;
return;
}
cout << "车辆信息如下:" << endl;
for (int i = 0; i < carList.size(); i++) {
cout << "品牌:" << carList[i].brand << endl;
cout << "型号:" << carList[i].model << endl;
cout << "生产年份:" << carList[i].year << endl;
cout << "颜色:" << carList[i].color << endl;
cout << "车牌号码:" << carList[i].licensePlate << endl;
cout << endl;
}
}
void modifyCar() {
if (carList.empty()) {
cout << "没有车辆信息!" << endl;
return;
}
string licensePlate;
cout << "请输入要修改的车牌号码:";
cin >> licensePlate;
bool found = false;
for (int i = 0; i < carList.size(); i++) {
if (carList[i].licensePlate == licensePlate) {
found = true;
cout << "请输入新的品牌:";
cin >> carList[i].brand;
cout << "请输入新的型号:";
cin >> carList[i].model;
cout << "请输入新的生产年份:";
cin >> carList[i].year;
cout << "请输入新的颜色:";
cin >> carList[i].color;
cout << "修改成功!" << endl;
break;
}
}
if (!found) {
cout << "未找到该车辆!" << endl;
}
}
void deleteCar() {
if (carList.empty()) {
cout << "没有车辆信息!" << endl;
return;
}
string licensePlate;
cout << "请输入要删除的车牌号码:";
cin >> licensePlate;
bool found = false;
for (vector<Car>::iterator it = carList.begin(); it != carList.end(); it++) {
if (it->licensePlate == licensePlate) {
found = true;
carList.erase(it);
cout << "删除成功!" << endl;
break;
}
}
if (!found) {
cout << "未找到该车辆!" << endl;
}
}
int main() {
while (true) {
cout << "请选择操作:1.添加车辆 2.查看车辆信息 3.修改车辆信息 4.删除车辆 5.退出" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
addCar();
break;
case 2:
viewCar();
break;
case 3:
modifyCar();
break;
case 4:
deleteCar();
break;
case 5:
return 0;
default:
cout << "无效操作!" << endl;
break;
}
}
}
```
该代码使用了一个结构体`Car`表示车辆信息,使用了`vector`容器存储多个车辆信息。在主函数中,使用了一个死循环,提供了添加车辆、查看车辆信息、修改车辆信息和删除车辆等操作。用户可以根据自己的需要进行选择。
阅读全文
相关推荐


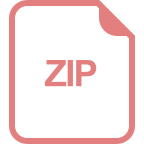
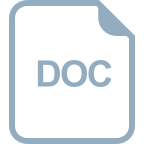
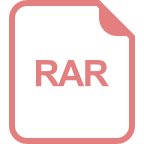
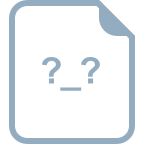
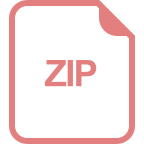
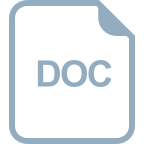
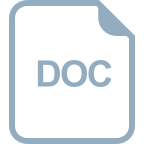
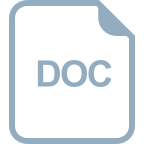
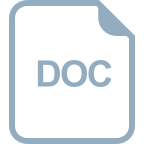
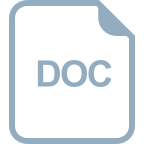
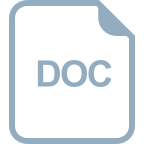
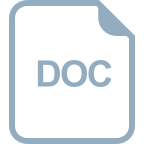
