初始条件:二叉树T存在,x是T中某个结点。 操作结果:若x是T的结点,则返回指向它的指针(查找成功,由于OJ的测试环境,显示TRUE);否则返回NULL(查找失败,显示FALSE)
时间: 2024-02-28 19:51:58 浏览: 40
这是一个二叉树的查找操作,可以使用递归或者迭代的方式来实现。以下是递归实现的伪代码:
```
TreeNode* search(TreeNode* root, int x) {
if (root == NULL || root->val == x) {
return root;
}
TreeNode* left = search(root->left, x);
if (left != NULL) {
return left;
}
return search(root->right, x);
}
```
其中,`root`是当前子树的根节点,`x`是要查找的节点的值。如果`root`为空或者`root`的值等于`x`,则直接返回`root`;否则递归查找左子树和右子树,如果找到了则返回找到的节点的指针,否则返回`NULL`表示查找失败。
注意,在实际实现中,需要根据具体情况来判断节点值的类型和比较方式。
相关问题
初始条件:二叉树T存在,x是T中某个结点。 操作结果:若x是T的结点,查找并返回指向x的兄弟结点的指针。 flag=0,返回左兄弟,若x是其双亲结点的左孩子或无左兄弟,则返回NULL。 flag=1,返回右兄弟,若x是其双亲结点的右孩子或无右兄弟,则返回NULL。写一个代码
以下是一份C++代码实现:
```C++
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x): val(x), left(NULL), right(NULL) {}
};
TreeNode* findSibling(TreeNode* root, TreeNode* x, int flag) {
if(!root || root == x) {
return NULL;
}
if(flag == 0) {
if(root->left == x) {
return NULL;
}
if(root->left && root->left != x) {
return root->left;
}
return findSibling(root->right, x, flag);
}
if(flag == 1) {
if(root->right == x) {
return NULL;
}
if(root->right && root->right != x) {
return root->right;
}
return findSibling(root->left, x, flag);
}
return NULL;
}
```
该函数接收三个参数:二叉树的根节点、需要查找兄弟节点的节点指针、以及flag的值。函数会递归地查找兄弟节点,并返回指向兄弟节点的指针。如果没有找到,则返回NULL。
建立线索二叉树,并实现:遍历线索二叉树,并在线索二叉树中插入结点和删除结点的操作。c语音
好的,我可以帮你实现这个功能。首先,我们需要定义一个结构体来表示线索二叉树的结点:
```c
typedef struct _threaded_node {
int data; // 结点的值
struct _threaded_node* left; // 左子树指针
struct _threaded_node* right; // 右子树指针
int ltag; // 左线索标记,0表示指向左子树,1表示指向前驱结点
int rtag; // 右线索标记,0表示指向右子树,1表示指向后继结点
} ThreadedNode;
```
这个结构体中,每个结点包括一个值、左子树指针、右子树指针和左右线索标记。
接下来,我们可以定义一个 `create_node` 函数,用于创建一个新的线索二叉树结点:
```c
ThreadedNode* create_node(int data) {
ThreadedNode* node = (ThreadedNode*) malloc(sizeof(ThreadedNode));
node->data = data;
node->left = NULL;
node->right = NULL;
node->ltag = 0;
node->rtag = 0;
return node;
}
```
这个函数接受一个值,然后创建一个新的线索二叉树结点,并将其初始化。注意,这个函数中我们将左右线索标记都设置为 `0`。
接下来,我们可以定义一个 `inorder_thread` 函数,用于将一个普通的二叉树转换为线索二叉树。这个函数采用中序遍历的方式遍历整个二叉树,并在遍历的过程中修改左右子树指针和线索标记:
```c
ThreadedNode* inorder_thread(ThreadedNode* root) {
ThreadedNode* prev = NULL;
ThreadedNode* curr = root;
ThreadedNode* head = create_node(0); // 头结点
head->ltag = 1; // 左线索标记为1,指向前驱结点
head->left = root; // 头结点的左子树指向根结点
while (curr != NULL) {
// 找到当前结点的前驱结点
while (curr->left != NULL && curr->ltag == 0) {
curr = curr->left;
}
// 将当前结点的左子树指针指向前驱结点
curr->left = prev;
curr->ltag = (prev == NULL) ? 1 : 0;
// 找到当前结点的后继结点
while (curr->right != NULL && curr->rtag == 0) {
curr = curr->right;
}
// 如果当前结点的右子树为空,则将其指向后继结点
if (curr->right == NULL) {
curr->right = prev;
curr->rtag = (prev == NULL) ? 1 : 0;
}
// 更新 prev 和 curr 指针
prev = curr;
curr = curr->right;
}
// 将头结点的右子树指针指向最后一个结点
prev->right = head;
prev->rtag = 1;
// 返回头结点
return head;
}
```
这个函数接受一个二叉树的根结点,然后返回一个新的线索二叉树的头结点。在遍历的过程中,我们用两个指针 `prev` 和 `curr` 分别表示前驱结点和当前结点。对于每个结点,我们首先找到它的前驱结点,然后将其左子树指针指向前驱结点,并将左线索标记设置为 `1`。然后,我们找到该结点的后继结点。如果该结点的右子树为空,则将其右子树指向后继结点,并将右线索标记设置为 `1`。最后,我们更新 `prev` 和 `curr` 指针,继续遍历下一个结点。遍历完成后,我们需要将头结点的右子树指针指向最后一个结点,并将右线索标记设置为 `1`。
接下来,我们可以定义一个 `inorder_traversal` 函数,用于中序遍历线索二叉树:
```c
void inorder_traversal(ThreadedNode* head) {
ThreadedNode* curr = head->left;
while (curr != head) {
while (curr->ltag == 0) {
curr = curr->left;
}
printf("%d ", curr->data);
while (curr->rtag == 1 && curr->right != head) {
curr = curr->right;
printf("%d ", curr->data);
}
curr = curr->right;
}
printf("\n");
}
```
这个函数接受一个线索二叉树的头结点,然后按照中序遍历的顺序遍历整个线索二叉树,并打印每个结点的值。在遍历的过程中,我们用一个指针 `curr` 表示当前结点。首先,我们找到第一个没有左子树的结点,并打印其值。然后,我们沿着右线索遍历到该结点的后继结点,并打印每个结点的值。最后,我们更新 `curr` 指针,继续遍历下一个结点,直到回到头结点。
最后,我们可以定义一个 `insert_node` 函数,用于在线索二叉树中插入一个新的结点。这个操作需要先将新结点插入到二叉树中,然后重新对二叉树进行线索化:
```c
void insert_node(ThreadedNode** root, int data) {
ThreadedNode* node = create_node(data);
if (*root == NULL) {
*root = node;
} else {
ThreadedNode* curr = *root;
ThreadedNode* parent = NULL;
while (curr != NULL) {
parent = curr;
if (data < curr->data) {
curr = curr->left;
} else {
curr = curr->right;
}
}
if (data < parent->data) {
parent->left = node;
} else {
parent->right = node;
}
}
*root = inorder_thread(*root);
}
```
这个函数接受一个指向根结点指针的指针和一个值,然后在线索二叉树中插入一个新的结点。如果根结点为空,则将新结点插入为根结点。否则,我们根据二叉搜索树的性质找到新结点应该插入的位置,并插入新结点。最后,我们重新对二叉树进行线索化。
类似地,我们也可以定义一个 `delete_node` 函数,用于在线索二叉树中删除一个结点。这个操作需要先将该结点从二叉树中删除,然后重新对二叉树进行线索化:
```c
void delete_node(ThreadedNode** root, int data) {
if (*root == NULL) {
return;
}
ThreadedNode* curr = *root;
ThreadedNode* parent = NULL;
while (curr != NULL && curr->data != data) {
parent = curr;
if (data < curr->data) {
curr = curr->left;
} else {
curr = curr->right;
}
}
if (curr == NULL) {
return;
}
if (curr->left == NULL && curr->right == NULL) { // 叶子结点
if (parent == NULL) {
*root = NULL;
} else if (curr == parent->left) {
parent->left = NULL;
} else {
parent->right = NULL;
}
free(curr);
} else if (curr->left == NULL) { // 只有右子树
if (parent == NULL) {
*root = curr->right;
} else if (curr == parent->left) {
parent->left = curr->right;
} else {
parent->right = curr->right;
}
free(curr);
} else if (curr->right == NULL) { // 只有左子树
if (parent == NULL) {
*root = curr->left;
} else if (curr == parent->left) {
parent->left = curr->left;
} else {
parent->right = curr->left;
}
free(curr);
} else { // 既有左子树又有右子树
ThreadedNode* min_node = curr->right;
while (min_node->left != NULL) {
min_node = min_node->left;
}
curr->data = min_node->data;
delete_node(&(curr->right), min_node->data);
}
*root = inorder_thread(*root);
}
```
这个函数接受一个指向根结点指针的指针和一个值,然后在线索二叉树中删除该值对应的结点。如果该值对应的结点是叶子结点,则直接删除。如果该结点只有左子树或右子树,则将其子树直接接到其父节点上。如果该结点既有左子树又有右子树,则先找到其右子树中的最小值,将其值复制到该结点中,然后递归删除右子树中的最小值结点。最后,我们重新对二叉树进行线索化。
以上就是一个简单的线索二叉树的实现,包括遍历线索二叉树、插入结点和删除结点等操作。你可以根据需要自行添加其他功能。
相关推荐
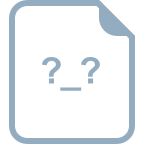












