import pandas as pd data_demo = [[1,2,3],[4,5,6],[7,8,9]] date_list = [datetime(2020,8,1),datetime(2020,8,1),datetime(2020,8,1)] time_df = pd.DataFra,e(data_demo,index=date_list) print(time_df)
时间: 2023-09-09 21:13:36 浏览: 55
I noticed that there is a typo in your code. Instead of `pd.DataFra,e`, it should be `pd.DataFrame`. Here is the corrected code:
```
import pandas as pd
from datetime import datetime
data_demo = [[1,2,3],[4,5,6],[7,8,9]]
date_list = [datetime(2020,8,1),datetime(2020,8,1),datetime(2020,8,1)]
time_df = pd.DataFrame(data_demo, index=date_list)
print(time_df)
```
This code creates a Pandas DataFrame called `time_df` with `data_demo` as the data and `date_list` as the index. The `datetime` module is used to create the `date_list`. Finally, the `print` statement outputs the contents of the `time_df` DataFrame.
相关问题
import pandas as pd import numpy as np path_marry=r"D:\迅雷下载\新建文件夹\数据集\数据可视化数据集\结婚离婚\结婚数据.csv" path_divorse=r"D:\迅雷下载\新建文件夹\数据集\数据可视化数据集\结婚离婚\离婚数据.csv" marry_data=pd.read_csv(path_marry) divorse_data=pd.read_csv(path_divorse) marry_data=marry_data.set_index(marry_data['地区']).drop(columns=["地区"]) #将索引变成地区 demo=marry_data.transpose() #transpose可以实现行列互换 demo["全国合计"]=demo.sum(axis=1) #沿着1轴相加 marry_data=demo.transpose() #取出要可视化的行列,一般索引为X轴数据,VALUE值为Y轴数据 show_data2=show_data2.sort_values() show_data2=marry_data["2019年"] show_data2=show_data2.iloc[:-1] x_axis_data=show_data2.index.tolist() y_axis_data=show_data2.values.tolist() from pyecharts import options as opts from pyecharts.charts import Bar from pyecharts.faker import Faker c = ( Bar(init_opts=opts.InitOpts(width="1000px",height="700px")) .add_xaxis(x_axis_data) .add_yaxis("2019年结婚登记数量(万对)", y_axis_data) .reversal_axis() #进行轴的转换 .set_series_opts(label_opts=opts.LabelOpts(position="right")) .set_global_opts(title_opts=opts.TitleOpts(title="全国2019年结婚登记数量(万对)")) ) c.render_notebook()为什么这个代码没有升序显示
在你修改代码时,将 `show_data2=show_data2.sort_values()` 放在了 `show_data2=marry_data["2019年"]` 的后面,这样的话排序并没有生效。你需要将这行代码放在读取数据和筛选数据之间,即在 `x_axis_data` 和 `y_axis_data` 定义之前:
```
import pandas as pd
import numpy as np
from pyecharts import options as opts
from pyecharts.charts import Bar
path_marry=r"D:\迅雷下载\新建文件夹\数据集\数据可视化数据集\结婚离婚\结婚数据.csv"
marry_data=pd.read_csv(path_marry)
marry_data=marry_data.set_index(marry_data['地区']).drop(columns=["地区"])
demo=marry_data.transpose()
demo["全国合计"]=demo.sum(axis=1)
marry_data=demo.transpose()
show_data2=marry_data["2019年"]
show_data2=show_data2.sort_values() # 将这行代码放在读取数据和筛选数据之间
show_data2=show_data2.iloc[:-1]
x_axis_data=show_data2.index.tolist()
y_axis_data=show_data2.values.tolist()
c = (
Bar(init_opts=opts.InitOpts(width="1000px",height="700px"))
.add_xaxis(x_axis_data)
.add_yaxis("2019年结婚登记数量(万对)", y_axis_data)
.reversal_axis()
.set_series_opts(label_opts=opts.LabelOpts(position="right"))
.set_global_opts(title_opts=opts.TitleOpts(title="全国2019年结婚登记数量(万对)"))
)
c.render_notebook()
```
这样的话,就可以升序排列展示了。
import json import pandas as pd import requests ur='https://v.api.aa1.cn/api/api-yq-all/index.php' headers={'user-agent':'Mozilla/5.0 (Windows NT 6.1; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/90.0.4430.212 Safari/537.36'} res=requests.get(url=ur,headers=headers).text dict=json.loads(res) lzt_list=dict['data']['areaTree'][2]['children'][8]['children'] name_list=[] lastUpdateTime_list=[] confirm_list=[] for i in lzt_list: name_list.append(i['name']) lastUpdateTime_list.append(i['lastUpdateTime']) confirm_list.append(i['total']['confirm']) #print(data_list,confirm_list,sep="\n") data=pd.DataFrame({"地点":name_list,"最后时间":lastUpdateTime_list,"感染人数":confirm_list}) print(name_list) print(lastUpdateTime_list) print(confirm_list) wc=pd.ExcelWriter("SeleniumDemo/海南疫情.xlsx") data.to_excel(wc,'data') wc._save() 解释这段代码的意
这段代码是一个 Python 程序,主要是从指定网址获取数据,解析数据,提取海南省各地市的疫情数据,然后将数据保存在 Excel 文件中。下面是具体的解释:
1. 首先,程序导入了三个模块:`json`、`pandas` 和 `requests`,分别用于处理 JSON 数据、操作数据框和发送 HTTP 请求。
2. 然后,程序定义了一个变量 `ur`,它存储了要获取数据的网址。
3. 接着,程序定义了一个请求头 `headers`,用于模拟浏览器向服务器发送请求。
4. 程序使用 `requests.get()` 方法发送 GET 请求,获取网页的响应内容,并使用 `text` 属性获取响应的文本内容。
5. 接下来,程序使用 `json.loads()` 方法将响应的 JSON 数据解析成 Python 字典类型。其中,`dict` 变量存储了整个字典数据。
6. 程序从字典中提取了海南省各地市的疫情数据,并将数据分别存储在 `name_list`、`lastUpdateTime_list` 和 `confirm_list` 三个列表中。
7. 然后,程序使用 `pd.DataFrame()` 方法将三个列表组合成一个数据框 `data`,其中,"地点"、"最后时间" 和 "感染人数" 分别是数据框的列名。
8. 最后,程序使用 `pd.ExcelWriter()` 方法创建一个 Excel 文件 `海南疫情.xlsx`,将数据框 `data` 存储在名为 "data" 的工作表中,并使用 `_save()` 方法将文件保存到本地磁盘中。
相关推荐
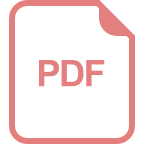
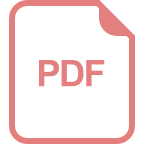












