list.remove() takes exactly one argument (2 given)
时间: 2023-05-26 11:02:34 浏览: 153
This error message is raised when the `remove()` method of a list object is called with more or less than one argument.
For example:
```
my_list = [1, 2, 3, 4]
my_list.remove() # Raises an error
```
In this example, `remove()` is called without any argument, which results in the error message because it requires one argument - the value to be removed.
On the other hand, if we pass more than one argument to `remove()`:
```
my_list = [1, 2, 3, 4]
my_list.remove(2, 3) # Raises an error
```
In this example, `remove()` is called with two arguments - `2` and `3`, which results in the error message because it requires only one argument - the value to be removed.
相关问题
list.append() takes exactly one argument (2 given)
这个错误消息的意思是,你在使用 `list.append()` 方法时给了它两个参数,但是这个方法只能接受一个参数。这意味着你应该只给 `list.append()` 方法传递一个参数。例如,如果你想向列表中添加一个新元素,你应该这样写:
```
my_list = [1, 2, 3]
my_list.append(4) # correct
```
而不是这样:
```
my_list = [1, 2, 3]
my_list.append(4, 5) # wrong - gives "TypeError: append() takes exactly one argument (2 given)"
```
TypeError: list.append() takes exactly one argument (2 given)
This error is raised when the `append()` method of a list is called with more than one argument. The `append()` method is used to add an element to the end of a list.
Example:
```
my_list = [1, 2, 3]
my_list.append(4, 5) # Raises TypeError: append() takes exactly one argument (2 given)
```
To fix this error, make sure that the `append()` method is called with only one argument.
Example:
```
my_list = [1, 2, 3]
my_list.append(4)
print(my_list) # Output: [1, 2, 3, 4]
```
相关推荐
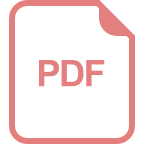
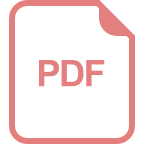
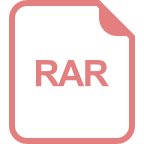












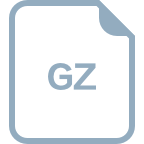