用C++读取一个有多列数据的txt文件,并把数据存入vector中
时间: 2024-05-13 22:16:36 浏览: 22
假设txt文件中每行数据格式为"列1 列2 列3 ...",其中每列数据之间用空格或制表符分隔,可以按照以下步骤读取并存入vector中:
1. 包含必要头文件
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <sstream>
```
2. 声明一个结构体或类来存储每行数据
```c++
struct Data {
int col1;
double col2;
std::string col3;
// ...其他列数据的类型和名称
};
```
3. 读取txt文件并解析每行数据
```c++
std::vector<Data> dataVec; // 定义存储数据的vector
std::ifstream inFile("data.txt"); // 打开txt文件
if (!inFile) {
std::cerr << "Failed to open file!" << std::endl;
return -1;
}
std::string line; // 存储每行数据的字符串
while (std::getline(inFile, line)) {
Data data; // 定义一个结构体对象来存储该行数据
std::istringstream iss(line); // 将该行数据转换为istringstream对象
iss >> data.col1 >> data.col2 >> data.col3; // 解析每列数据并存入结构体对象中
// ...解析其他列数据并存入结构体对象中
dataVec.push_back(data); // 将该行数据的结构体对象存入vector中
}
```
4. 关闭文件并使用vector中的数据
```c++
inFile.close(); // 关闭文件
// 使用vector中的数据,例如输出第一行第二列数据
std::cout << "The second column of the first row is: " << dataVec[0].col2 << std::endl;
```
完整代码如下:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <sstream>
struct Data {
int col1;
double col2;
std::string col3;
// ...其他列数据的类型和名称
};
int main() {
std::vector<Data> dataVec; // 定义存储数据的vector
std::ifstream inFile("data.txt"); // 打开txt文件
if (!inFile) {
std::cerr << "Failed to open file!" << std::endl;
return -1;
}
std::string line; // 存储每行数据的字符串
while (std::getline(inFile, line)) {
Data data; // 定义一个结构体对象来存储该行数据
std::istringstream iss(line); // 将该行数据转换为istringstream对象
iss >> data.col1 >> data.col2 >> data.col3; // 解析每列数据并存入结构体对象中
// ...解析其他列数据并存入结构体对象中
dataVec.push_back(data); // 将该行数据的结构体对象存入vector中
}
inFile.close(); // 关闭文件
// 使用vector中的数据,例如输出第一行第二列数据
std::cout << "The second column of the first row is: " << dataVec[0].col2 << std::endl;
return 0;
}
```
相关推荐
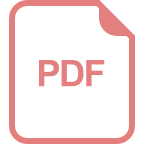
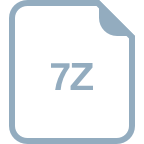














