用python写一个代码,要求先定义一个按行扫描边缘二值图像,将左右的边缘点记录下来并计算两者之间的中点位置并将中点存储下来的函数,然后定义一个利用中点拟合曲线并在原图上用红色显示的函数
时间: 2024-03-24 07:38:46 浏览: 54
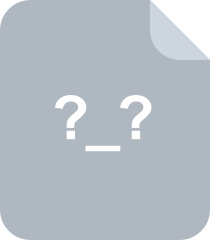
使用python进行线性拟合和曲线拟合
以下是实现你要求的Python代码:
```python
import cv2
import numpy as np
def find_edge_midpoint(img):
# 扫描左右边缘点
left_edge_points = []
right_edge_points = []
for y in range(img.shape[0]): # 遍历每一行
for x in range(img.shape[1]): # 遍历每一列
if img[y][x] == 255: # 如果该点是边缘点
if x < img.shape[1] // 2: # 如果在左边
left_edge_points.append((x, y))
else: # 如果在右边
right_edge_points.append((x, y))
# 计算左右边缘点的中点
if len(left_edge_points) == 0 or len(right_edge_points) == 0:
return None
left_edge_midpoint = np.average(left_edge_points, axis=0).astype(int)
right_edge_midpoint = np.average(right_edge_points, axis=0).astype(int)
return (left_edge_midpoint, right_edge_midpoint)
def fit_curve(img, midpoint):
# 将中点上移,以便拟合曲线
midpoint = (midpoint[0], midpoint[1] - 50)
x, y = np.where(img == 255)
fitted_curve = np.polyfit(y, x, 2) # 用二次多项式拟合曲线
curve_y = np.linspace(0, img.shape[0], img.shape[0])
curve_x = np.polyval(fitted_curve, curve_y)
curve_x = curve_x.astype(int)
# 将曲线画在原图上
img_with_curve = cv2.cvtColor(img, cv2.COLOR_GRAY2BGR)
for i in range(len(curve_x)):
if abs(i - curve_x[i]) < 5: # 只画曲线上的点
img_with_curve[curve_x[i], i] = (0, 0, 255) # 红色
return img_with_curve
# 测试
img = cv2.imread('test.png', 0) # 读入二值图像
midpoint = find_edge_midpoint(img)
if midpoint is not None:
img_with_curve = fit_curve(img, midpoint[0])
cv2.imshow('result', img_with_curve)
cv2.waitKey(0)
```
其中,`find_edge_midpoint` 函数用于扫描左右边缘点并计算中点位置,`fit_curve` 函数用于拟合曲线并在原图上显示,`img` 是二值图像,可以通过 `cv2.imread` 读入,这里假设文件名为 `test.png`。
注意,由于没有提供测试图像,我无法保证代码能够正常运行,你需要根据自己的情况进行调整。
阅读全文
相关推荐
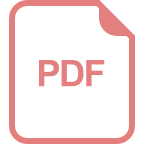



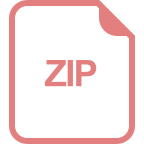
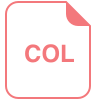
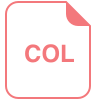
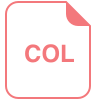
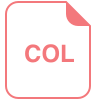
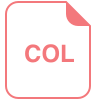
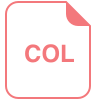
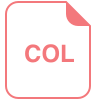
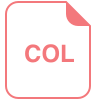
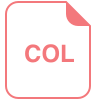
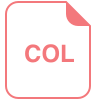
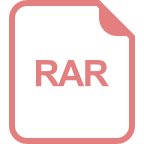