dlib c++ 人脸识别 使用GPU加速, 需要多线程处理多个图像,需要将图像处理流程封装成函数或类;要求实时进行人脸识别,要求对程序进行优化以提高识别速度。要求更加精细的多线程处理方式和更加高级的优化算法
时间: 2024-01-24 19:18:58 浏览: 159
针对你的需求,我可以提供以下建议:
1. 将图像处理流程封装成函数或类:将人脸识别的图像处理流程封装成函数或类,可以使代码更加模块化和可读性更高。
2. 使用多线程处理多个图像:使用多线程可以充分利用 CPU 处理能力,提高人脸识别速度。可以考虑使用 OpenMP 或者 C++11 提供的 std::thread 来实现。
3. 使用 GPU 加速:使用 GPU 可以大大提高人脸识别的速度。可以使用 Nvidia 提供的 CUDA 或者 OpenCL 来实现。
4. 优化算法:对于人脸识别算法进行优化可以提高识别速度。可以考虑使用更加高效的特征提取算法,如 LBP 特征、Haar 特征或者深度学习算法。
5. 精细的多线程处理方式:可以根据处理图像的数量、图像的大小和 CPU 核心数等因素,采用不同的多线程方式。比如使用线程池来避免线程创建销毁的开销、使用任务队列来避免线程竞争等。
6. 高级的优化算法:可以使用更加高级的优化算法,如 Intel MKL 库提供的 BLAS、FFT 等数学库,或者使用深度学习框架来进行人脸识别。
相关问题
dlib c++ 人脸识别 使用GPU加速, 需要多线程处理多个图像,需要将图像处理流程封装成函数或类;需要实时进行人脸识别,需要对程序进行优化以提高识别速度。
针对您的需求,可以将图像处理流程封装成一个类,类中包含多个线程,每个线程处理一个图像。为了提高识别速度,可以使用GPU加速,使用dlib库提供的cuda相关函数进行加速。
以下是一个简单的示例代码:
```c++
#include <iostream>
#include <thread>
#include <vector>
#include <dlib/opencv.h>
#include <opencv2/opencv.hpp>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing.h>
#include <dlib/cuda/cuda_dlib.h>
using namespace std;
using namespace dlib;
class FaceDetector {
public:
FaceDetector() {
detector = get_frontal_face_detector();
cuda::set_dlib_cuda_enabled();
}
void detect(cv::Mat& image) {
cv_image<bgr_pixel> cimg(image);
matrix<rgb_pixel> img;
assign_image(img, cimg);
std::vector<rectangle> faces;
// Run the face detector on the image of our action hero.
faces = detector(img);
// Print the number of faces found in the image
std::cout << "Number of faces detected: " << faces.size() << std::endl;
// Draw a rectangle for each face
for (unsigned long i = 0; i < faces.size(); ++i) {
rectangle r(faces[i].left(), faces[i].top(), faces[i].right(), faces[i].bottom());
cv::rectangle(image, cv::Point(r.left(), r.top()), cv::Point(r.right(), r.bottom()), cv::Scalar(0, 255, 0), 2);
}
}
private:
frontal_face_detector detector;
};
int main() {
cv::VideoCapture cap(0);
FaceDetector fd;
while (true) {
cv::Mat frame;
cap >> frame;
fd.detect(frame);
cv::imshow("face detection", frame);
cv::waitKey(1);
}
return 0;
}
```
在这个示例代码中,我们使用了dlib库提供的前置人脸检测器 `get_frontal_face_detector()` 进行人脸检测,使用了OpenCV库进行图像读取与显示。我们将人脸检测封装成了一个类 `FaceDetector`,其中包含了一个 `detect()` 函数,用于对传入的图像进行人脸检测。在主函数中,我们通过调用 `detect()` 函数对摄像头捕获到的图像进行人脸检测,并将检测结果显示出来。
为了使用GPU加速,我们使用了 `dlib/cuda/cuda_dlib.h` 头文件中提供的 `set_dlib_cuda_enabled()` 函数来启用CUDA支持。这样,在进行人脸检测时,dlib库会自动使用GPU进行加速。
当然,如果您的需求更加复杂,可能需要更加精细的多线程处理方式和更加高级的优化算法。但是这个示例代码可以作为一个简单的参考,供您进行参考。
dlib c++ 人脸识别 详细例子, 需要处理多个图像,则需要将图像处理流程封装成函数或类;需要实时进行人脸识别,需要对程序进行优化以提高识别速度。
以下是一个简单的dlib C++人脸识别示例,包括人脸检测和人脸特征提取:
```c++
#include <dlib/opencv.h>
#include <dlib/image_processing.h>
#include <dlib/image_processing/frontal_face_detector.h>
#include <dlib/image_processing/render_face_detections.h>
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
using namespace dlib;
int main()
{
// 初始化人脸检测器
frontal_face_detector detector = get_frontal_face_detector();
// 初始化人脸特征提取器
shape_predictor sp;
deserialize("shape_predictor_68_face_landmarks.dat") >> sp;
// 加载图像
cv::Mat img = cv::imread("test.jpg");
// 将OpenCV图像转换为dlib图像
dlib::cv_image<dlib::bgr_pixel> dlib_img(img);
// 检测人脸
std::vector<dlib::rectangle> dets = detector(dlib_img);
// 循环遍历每个检测到的人脸
for (unsigned long i = 0; i < dets.size(); ++i)
{
// 提取人脸特征
full_object_detection shape = sp(dlib_img, dets[i]);
// 在图像上绘制人脸特征点
for (unsigned long i = 0; i < shape.num_parts(); ++i)
{
cv::circle(img, cv::Point(shape.part(i).x(), shape.part(i).y()), 2, cv::Scalar(0, 0, 255), -1);
}
// 在图像上绘制人脸框
cv::rectangle(img, cv::Point(dets[i].left(), dets[i].top()), cv::Point(dets[i].right(), dets[i].bottom()), cv::Scalar(0, 255, 0), 2);
}
// 显示图像
cv::imshow("result", img);
cv::waitKey(0);
return 0;
}
```
如果需要处理多个图像,可以将上述代码封装成一个函数或类,并在外部循环中调用该函数或类。
如果需要实时进行人脸识别,可以对程序进行优化,例如使用多线程处理图像、使用GPU加速等。另外,dlib也提供了一些用于优化人脸识别速度的技术,例如使用人脸姿势估计来加速人脸特征提取。
阅读全文
相关推荐
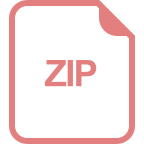


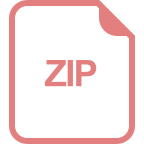
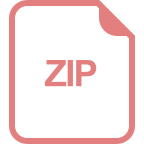
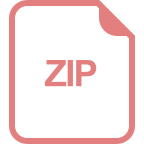
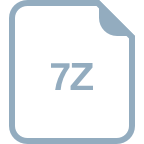
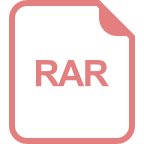
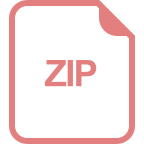
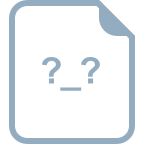
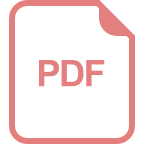
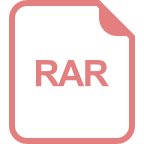
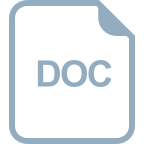
