C++程序实现kalman滤波观测值逐渐更新的扩展的kalman滤波计算源代码
时间: 2024-04-08 08:34:25 浏览: 60
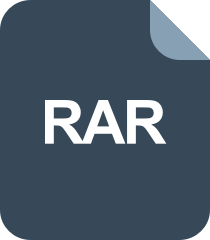
一个扩展卡尔曼滤波程序
以下是一个简单的C++程序实现扩展卡尔曼滤波(Extended Kalman Filter,EKF)的观测值逐渐更新的计算源代码示例:
```cpp
#include <iostream>
#include <cmath>
#include <Eigen/Dense>
using namespace std;
using namespace Eigen;
// 状态转移函数
VectorXd stateTransitionFunction(const VectorXd& x)
{
VectorXd x_new(2);
x_new(0) = x(0) + 1;
x_new(1) = x(1) + 2;
return x_new;
}
// 观测函数
VectorXd observationFunction(const VectorXd& x)
{
VectorXd z(1);
z(0) = sin(x(0));
return z;
}
// 扩展卡尔曼滤波算法
void extendedKalmanFilter(VectorXd& x, MatrixXd& P, const VectorXd& z)
{
// 预测步骤
VectorXd x_pred = stateTransitionFunction(x);
MatrixXd F(2, 2);
F << 1, 0, 0, 1; // 状态转移矩阵的雅可比矩阵
MatrixXd P_pred = F * P * F.transpose();
// 更新步骤
VectorXd z_pred = observationFunction(x_pred);
VectorXd y = z - z_pred;
MatrixXd H(1, 2);
H << cos(x_pred(0)), 0; // 观测函数的雅可比矩阵
MatrixXd S = H * P_pred * H.transpose() + MatrixXd::Identity(1, 1); // 测量噪声协方差矩阵
MatrixXd K = P_pred * H.transpose() * S.inverse();
x = x_pred + K * y;
P = (MatrixXd::Identity(2, 2) - K * H) * P_pred;
}
int main()
{
// 初始状态估计和协方差矩阵
VectorXd x(2);
x << 0, 0; // 初始状态估计
MatrixXd P(2, 2);
P << 1, 0, 0, 1; // 初始协方差矩阵
// 观测值
VectorXd z(1);
z << 0.5;
// 逐步更新观测值
for (int i = 0; i < 10; ++i)
{
extendedKalmanFilter(x, P, z);
cout << "Updated state estimate: " << x.transpose() << endl;
}
return 0;
}
```
这个示例程序展示了一个简单的扩展卡尔曼滤波算法,其中包括状态转移函数和观测函数的定义,以及扩展卡尔曼滤波的预测和更新步骤。在主函数中,我们逐步更新观测值,并输出每次更新后的状态估计结果。请注意,这只是一个简单的示例,实际使用时可能需要根据具体问题进行适当的修改。
阅读全文
相关推荐
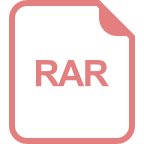
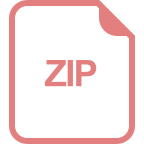
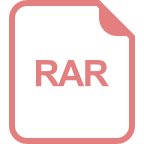
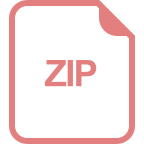
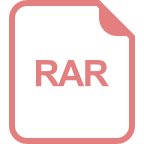
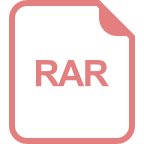
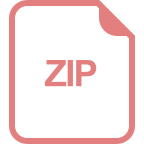
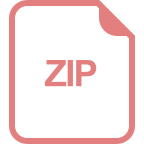
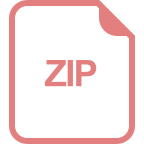
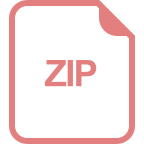
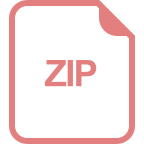
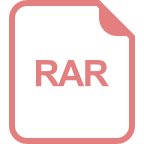
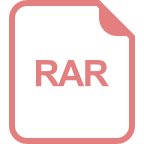
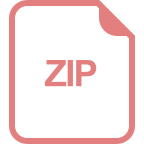
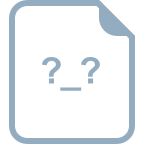
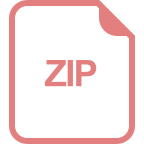
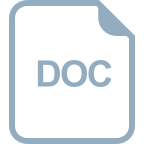