c++ bfs和dfs算法模板
时间: 2023-08-29 11:12:30 浏览: 148
BFS(广度优先搜索)算法模板:
```c++
#include <iostream>
#include <queue>
using namespace std;
const int N = 1e5 + 10;
int n; // 图的大小
int g[N][N]; // 存储图的邻接矩阵
bool st[N]; // 标记数组
void bfs(int u) {
queue<int> q;
q.push(u);
st[u] = true;
while (!q.empty()) {
int t = q.front();
q.pop();
// 处理节点 t
for (int i = 1; i <= n; i++) {
if (g[t][i] && !st[i]) {
q.push(i);
st[i] = true;
}
}
}
}
int main() {
// 读入图
cin >> n;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
cin >> g[i][j];
}
}
// BFS 遍历整张图
for (int i = 1; i <= n; i++) {
if (!st[i]) bfs(i);
}
return 0;
}
```
DFS(深度优先搜索)算法模板:
```c++
#include <iostream>
using namespace std;
const int N = 1e5 + 10;
int n; // 图的大小
int g[N][N]; // 存储图的邻接矩阵
bool st[N]; // 标记数组
void dfs(int u) {
st[u] = true;
// 处理节点 u
for (int i = 1; i <= n; i++) {
if (g[u][i] && !st[i]) {
dfs(i);
}
}
}
int main() {
// 读入图
cin >> n;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= n; j++) {
cin >> g[i][j];
}
}
// DFS 遍历整张图
for (int i = 1; i <= n; i++) {
if (!st[i]) dfs(i);
}
return 0;
}
```
注意:以上模板都是针对无向图的,如果是有向图需要修改邻接矩阵的定义和遍历方式。
阅读全文
相关推荐
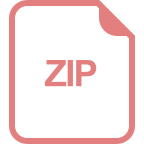

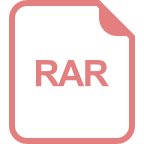
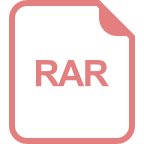
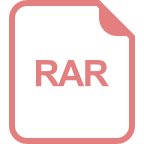
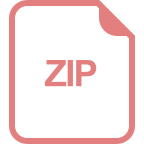
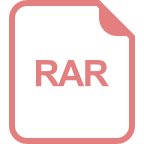
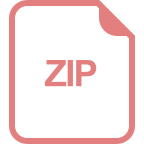
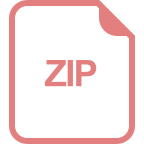
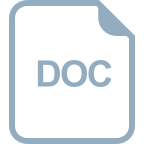
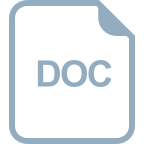

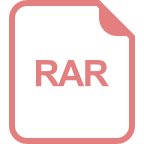
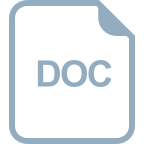
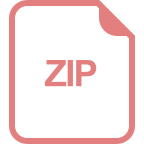
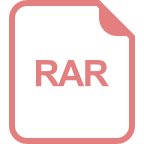
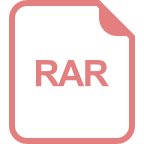