将包含有学生学号、姓名、性别、专业、联系方式等信息的若干学生对象保存到文件stud.dat中,要求保密联系方式(即不保存),然后再读取文件中的学生信息并按学号的升序输出,用java编程实现
时间: 2024-03-19 09:39:58 浏览: 23
好的,这是一个比较典型的文件读写问题,我们可以通过Java的输入输出流来实现。具体实现方法如下:
1.创建一个Student类,包含学号、姓名、性别、专业、联系方式等属性,并添加Getter和Setter方法。
```
public class Student {
private String id; // 学号
private String name; // 姓名
private String gender; // 性别
private String major; // 专业
private String contact; // 联系方式
// Getter和Setter方法省略
}
```
2.创建一个文件保存和读取的工具类FileUtil,包含两个方法:writeToFile()和readFromFile()。
```
import java.io.*;
public class FileUtil {
// 将学生信息保存到文件
public static void writeToFile(Student[] students, String fileName) {
try {
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(fileName));
for (Student student : students) {
// 将Student对象写入文件
oos.writeObject(student);
}
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
// 从文件中读取学生信息
public static Student[] readFromFile(String fileName) {
Student[] students = new Student[10]; // 假设最多有10个学生
int count = 0; // 统计实际读取到的学生数量
try {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(fileName));
while (true) {
// 从文件中读取Student对象
Student student = (Student) ois.readObject();
if (student != null) {
student.setContact(""); // 不保存联系方式
students[count++] = student;
} else {
break;
}
}
ois.close();
} catch (EOFException e) {
// 文件读取完毕
} catch (IOException | ClassNotFoundException e) {
e.printStackTrace();
}
return students;
}
}
```
3.在主函数中创建若干个学生对象,并使用FileUtil.writeToFile()将学生信息保存到文件stud.dat中。
```
public static void main(String[] args) {
Student[] students = new Student[3];
students[0] = new Student("001", "张三", "男", "计算机科学与技术", "13333333333");
students[1] = new Student("002", "李四", "女", "软件工程", "14444444444");
students[2] = new Student("003", "王五", "男", "信息安全", "15555555555");
FileUtil.writeToFile(students, "stud.dat");
}
```
4.在主函数中使用FileUtil.readFromFile()从文件中读取学生信息,并按学号的升序输出。
```
public static void main(String[] args) {
// 保存学生信息到文件
Student[] students = new Student[3];
students[0] = new Student("001", "张三", "男", "计算机科学与技术", "13333333333");
students[1] = new Student("002", "李四", "女", "软件工程", "14444444444");
students[2] = new Student("003", "王五", "男", "信息安全", "15555555555");
FileUtil.writeToFile(students, "stud.dat");
// 从文件中读取学生信息并按学号的升序输出
students = FileUtil.readFromFile("stud.dat");
Arrays.sort(students, Comparator.comparing(Student::getId));
for (Student student : students) {
if (student != null) {
System.out.println(student.getId() + " " + student.getName() + " " + student.getGender() + " " + student.getMajor());
}
}
}
```
这样就完成了将学生信息保存到文件、读取文件中的学生信息并按学号的升序输出的需求。
相关推荐
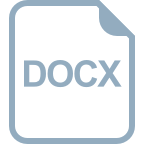



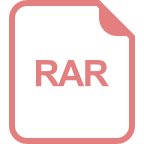
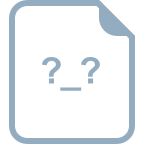
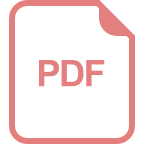
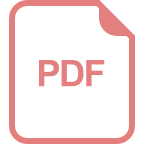
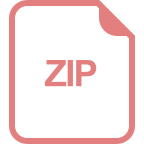