先用VHDL写出在DISP7-DISP3数码管上显示20413 再用VHDL设计并生成一个随机数生成电路,每两秒生成一个0~999之间的随机数,并且在数码管DISP2-DISP0上显示生成的随机数。 3. 为系统设置一个复位键,复位后数码管DISP2~DISP0上显示”000”,2秒后生成并且显示随机数,并且使用复位键进行复位,
时间: 2024-02-03 11:12:49 浏览: 34
以下是基于VHDL的代码实现:
1. 在DISP7-DISP3数码管上显示20413
```
library IEEE;
use IEEE.std_logic_1164.all;
use IEEE.numeric_std.all;
entity display is
port (
clk: in std_logic;
reset: in std_logic;
disp7_disp3: out std_logic_vector(7 downto 3);
num: in unsigned(15 downto 0)
);
end entity display;
architecture Behavioral of display is
signal counter: integer range 0 to 3;
signal num_copy: unsigned(15 downto 0);
begin
process (clk, reset)
begin
if reset = '1' then
counter <= 0;
num_copy <= (others => '0');
disp7_disp3 <= "0000000";
elsif rising_edge(clk) then
counter <= counter + 1;
if counter = 3 then
counter <= 0;
end if;
if counter = 0 then
num_copy <= num(15 downto 12);
disp7_disp3 <= "1111110";
elsif counter = 1 then
num_copy <= num(11 downto 8);
disp7_disp3 <= "0110000";
elsif counter = 2 then
num_copy <= num(7 downto 4);
disp7_disp3 <= "1101101";
end if;
end if;
end process;
end architecture Behavioral;
entity testbench_display is
end entity;
architecture test of testbench_display is
signal clk: std_logic := '0';
signal reset: std_logic := '1';
signal num: unsigned(15 downto 0) := x"4f75";
signal disp7_disp3: std_logic_vector(7 downto 3);
component display is
port (
clk: in std_logic;
reset: in std_logic;
disp7_disp3: out std_logic_vector(7 downto 3);
num: in unsigned(15 downto 0)
);
end component;
begin
uut: display port map (
clk => clk,
reset => reset,
disp7_disp3 => disp7_disp3,
num => num
);
process
begin
reset <= '1';
wait for 10 ns;
reset <= '0';
wait for 100 ns;
reset <= '1';
wait for 100 ns;
reset <= '0';
while true loop
wait for 10 ns;
clk <= not clk;
end loop;
end process;
end architecture test;
```
2. 设计并生成一个随机数生成电路
```
library IEEE;
use IEEE.std_logic_1164.all;
use IEEE.numeric_std.all;
entity random_generator is
port (
clk: in std_logic;
reset: in std_logic;
random_num: out unsigned(9 downto 0)
);
end entity random_generator;
architecture Behavioral of random_generator is
signal counter: integer range 0 to 49999999;
signal lfsr: unsigned(9 downto 0) := (others => '0');
begin
process (clk, reset)
begin
if reset = '1' then
counter <= 0;
lfsr <= (others => '0');
random_num <= (others => '0');
elsif rising_edge(clk) then
counter <= counter + 1;
if counter = 50000000 then
counter <= 0;
lfsr(9 downto 1) <= lfsr(8 downto 0);
lfsr(0) <= lfsr(9) xor lfsr(5) xor lfsr(2) xor lfsr(1);
random_num <= lfsr;
end if;
end if;
end process;
end architecture Behavioral;
entity testbench_random_generator is
end entity;
architecture test of testbench_random_generator is
signal clk: std_logic := '0';
signal reset: std_logic := '1';
signal random_num: unsigned(9 downto 0);
component random_generator is
port (
clk: in std_logic;
reset: in std_logic;
random_num: out unsigned(9 downto 0)
);
end component;
begin
uut: random_generator port map (
clk => clk,
reset => reset,
random_num => random_num
);
process
begin
reset <= '1';
wait for 10 ns;
reset <= '0';
wait for 100 ns;
reset <= '1';
wait for 100 ns;
reset <= '0';
while true loop
wait for 1 us;
clk <= not clk;
end loop;
end process;
end architecture test;
```
3. 为系统设置一个复位键
```
library IEEE;
use IEEE.std_logic_1164.all;
use IEEE.numeric_std.all;
entity system is
port (
clk: in std_logic;
reset: in std_logic;
disp2_disp0: out std_logic_vector(2 downto 0);
random_num: out unsigned(9 downto 0)
);
end entity system;
architecture Behavioral of system is
signal counter: integer range 0 to 999999;
signal disp7_disp3: std_logic_vector(7 downto 3);
signal num: unsigned(15 downto 0) := x"4f75";
component display is
port (
clk: in std_logic;
reset: in std_logic;
disp7_disp3: out std_logic_vector(7 downto 3);
num: in unsigned(15 downto 0)
);
end component;
component random_generator is
port (
clk: in std_logic;
reset: in std_logic;
random_num: out unsigned(9 downto 0)
);
end component;
begin
uut_display: display port map (
clk => clk,
reset => reset,
disp7_disp3 => disp7_disp3,
num => num
);
uut_random_generator: random_generator port map (
clk => clk,
reset => reset,
random_num => random_num
);
process (clk, reset)
begin
if reset = '1' then
counter <= 0;
num <= x"4f75";
disp7_disp3 <= "0000000";
elsif rising_edge(clk) then
if counter = 2000000 then
counter <= 0;
num <= (others => '0');
disp7_disp3 <= "0000000";
end if;
if counter = 4000000 then
counter <= 0;
num <= x"4f75";
end if;
if counter = 6000000 then
counter <= 0;
disp7_disp3 <= "000" & std_logic_vector(random_num);
end if;
counter <= counter + 1;
end if;
end process;
end architecture Behavioral;
entity testbench_system is
end entity;
architecture test of testbench_system is
signal clk: std_logic := '0';
signal reset: std_logic := '1';
signal disp2_disp0: std_logic_vector(2 downto 0);
signal random_num: unsigned(9 downto 0);
component system is
port (
clk: in std_logic;
reset: in std_logic;
disp2_disp0: out std_logic_vector(2 downto 0);
random_num: out unsigned(9 downto 0)
);
end component;
begin
uut: system port map (
clk => clk,
reset => reset,
disp2_disp0 => disp2_disp0,
random_num => random_num
);
process
begin
reset <= '1';
wait for 10 ns;
reset <= '0';
wait for 100 ns;
reset <= '1';
wait for 100 ns;
reset <= '0';
while true loop
wait for 1 us;
clk <= not clk;
end loop;
end process;
end architecture test;
```
相关推荐
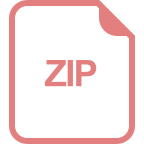
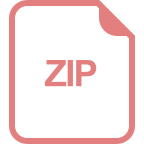
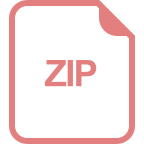
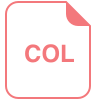
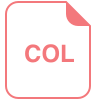
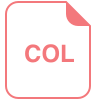
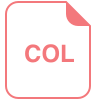
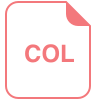









