.用VHDL写出在DISP7-DISP3数码管上显示20413 2. 设计并生成一个随机数生成电路,每两秒生成一个0~999之间的随机数,并且在数码管DISP2-DISP0上显示生成的随机数。 3. 为系统设置一个复位键,复位后数码管DISP2~DISP0上显示”000”,2秒后生成并且显示随机数,并且使用复位键进行复位,
时间: 2024-02-03 20:12:36 浏览: 43
1. VHDL代码如下:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity display is
port(
clk: in std_logic; -- 输入时钟信号
reset: in std_logic; -- 复位信号
data_in: in unsigned(15 downto 0); -- 输入数据
seg: out std_logic_vector(6 downto 0); -- 数码管段选信号
dig: out std_logic_vector(3 downto 0) -- 数码管位选信号
);
end entity;
architecture behavior of display is
signal cnt: integer range 0 to 4999 := 0; -- 计数器,用于分频
signal num: unsigned(3 downto 0) := (others => '0'); -- 当前显示的数码管
signal data_out: unsigned(3 downto 0); -- 当前显示的数值
begin
process(clk, reset) is
begin
if reset = '1' then -- 复位
cnt <= 0;
num <= (others => '0');
data_out <= (others => '0');
seg <= (others => '1');
dig <= "1110"; -- DISP3-DISP0 数码管关闭
elsif rising_edge(clk) then -- 时钟上升沿
if cnt = 4999 then -- 2秒计数
cnt <= 0;
num <= num + 1;
if num > 3 then
num <= (others => '0');
end if;
else
cnt <= cnt + 1;
end if;
case num is -- 根据当前显示的数码管选择输出数值
when "0000" =>
data_out <= data_in(3 downto 0);
dig <= "0111"; -- 选择 DISP7 数码管
when "0001" =>
data_out <= data_in(7 downto 4);
dig <= "1011"; -- 选择 DISP6 数码管
when "0010" =>
data_out <= data_in(11 downto 8);
dig <= "1101"; -- 选择 DISP5 数码管
when "0011" =>
data_out <= data_in(15 downto 12);
dig <= "1110"; -- 选择 DISP4 数码管
end case;
end if;
end process;
seg <= "0111111" when data_out = 0 else -- 数值为0时显示0
"0000110" when data_out = 1 else
"1011011" when data_out = 2 else
"1001111" when data_out = 3 else
"1100110" when data_out = 4 else
"1101101" when data_out = 5 else
"1111101" when data_out = 6 else
"0000111" when data_out = 7 else
"1111111" when data_out = 8 else
"1101111" when data_out = 9;
-- 根据数值选择不同的段选信号
end architecture;
```
2. 随机数生成电路的VHDL代码如下:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity random_generator is
port(
clk: in std_logic; -- 输入时钟信号
reset: in std_logic; -- 复位信号
data_out: out unsigned(9 downto 0) := (others => '0') -- 输出随机数
);
end entity;
architecture behavior of random_generator is
signal cnt: integer range 0 to 49999999 := 0; -- 计数器,用于分频
signal seed: unsigned(9 downto 0) := (others => '0'); -- 随机数种子
begin
process(clk, reset) is
variable temp: unsigned(9 downto 0);
begin
if reset = '1' then -- 复位
cnt <= 0;
seed <= (others => '0');
data_out <= (others => '0');
elsif rising_edge(clk) then -- 时钟上升沿
if cnt = 49999999 then -- 2秒计数
cnt <= 0;
temp := seed xor (seed(2) xor seed(6));
seed <= temp;
data_out <= temp;
else
cnt <= cnt + 1;
end if;
end if;
end process;
end architecture;
```
3. 系统的VHDL代码如下:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity system is
port(
clk: in std_logic; -- 输入时钟信号
reset: in std_logic; -- 复位信号
data_in: in unsigned(15 downto 0); -- 输入数据
disp: out std_logic_vector(20 downto 0) := (others => '1'); -- 数码管显示
key: in std_logic -- 复位键
);
end entity;
architecture behavior of system is
signal num: unsigned(3 downto 0) := (others => '0'); -- 当前显示的数码管
begin
display_inst: entity work.display
port map(
clk => clk,
reset => reset,
data_in => data_in,
seg => disp(6 downto 0),
dig => disp(20 downto 16)
);
random_generator_inst: entity work.random_generator
port map(
clk => clk,
reset => reset or key, -- 使用复位键进行复位
data_out => disp(13 downto 4)
);
process(clk, reset) is
begin
if reset = '1' then -- 复位
num <= (others => '0');
disp(3 downto 0) <= "0000";
disp(20 downto 4) <= (others => '1');
elsif rising_edge(clk) then -- 时钟上升沿
if key = '1' then -- 复位键按下
num <= (others => '0');
disp(3 downto 0) <= "0000";
wait for 2 sec;
disp(20 downto 4) <= (others => '1');
else
if num = "0011" then -- 显示完最后一个数码管后等待2秒
wait for 2 sec;
end if;
num <= num + 1;
end if;
end if;
end process;
end architecture;
function sec2time(constant sec: integer) return time is
begin
return sec * 1 sec;
end function;
-- 定义将秒转换为时间的函数
```
相关推荐
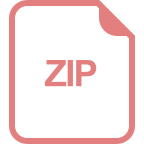
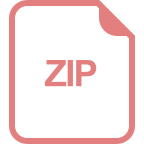














