interface Com { int add(int a,int b); } abstract class A { abstract int add(int a,int b); } class B extends A implements Com{ public int add(int a,int b) { return a+b; } } public class E { public static void main(String args[]) { B b = new B(); Com com = b; System.out.println(com.add(12,6)); //【代码1】 A a = b; System.out.println(a.add(10,5)); //【代码2】 } }注释翻译上面的代码
时间: 2024-04-04 17:07:27 浏览: 37
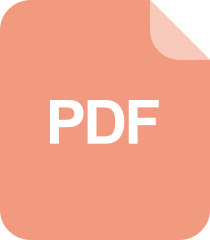
美团点评2017秋招笔试真题-前端开发工程师A.pdf
这段代码定义了一个接口`Com`和一个抽象类`A`,并且一个具体类`B`继承了`A`并实现了`Com`接口。在`E`类的`main()`方法中,创建了一个`B`类的实例`b`,并将它赋值给了`Com`类型的变量`com`和`A`类型的变量`a`。在`【代码1】`和`【代码2】`处分别调用了`com.add(12,6)`和`a.add(10,5)`方法,并输出了它们的返回值。由于`B`类中实现了`add()`方法,因此这两个方法的返回值都是`18`。
阅读全文
相关推荐
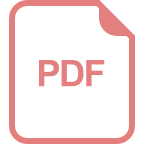
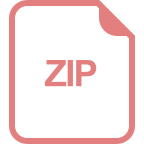

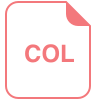
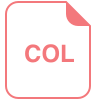
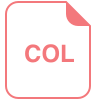
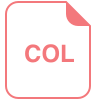
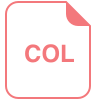
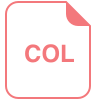
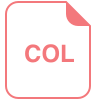
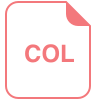





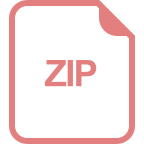
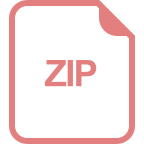
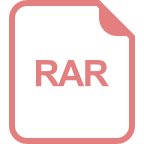