在上述条件下void display_person(struct person p[],int n, int flag);输出每个公民信息的函数。其中数组 p 存放 n 个公民信息。当参数flag=1时,输出正确公民信息和人数,当flag=0时,输出错误公民信息和人数。
时间: 2024-03-31 20:36:44 浏览: 61
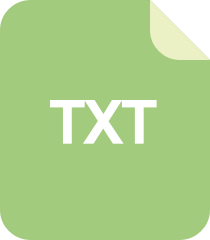
设计一个人类person,包括人员编号和姓名等数据成员以及相关的成员函数
可以使用以下代码输出每个公民信息的函数:
```c++
#include <string.h>
#include <stdio.h>
#include <stdlib.h>
struct birth { int year, month, day; };
struct person {char ID[19], name[20]; struct birth birthday; int flag; };
int checkID(char id[]) {
int i, sum = 0;
int weight[] = {7, 9, 10, 5, 8, 4, 2, 1, 6, 3, 7, 9, 10, 5, 8, 4, 2};
char check_code[] = "10X98765432";
for (i = 0; i < 17; i++) {
sum += (id[i] - '0') * weight[i];
}
int mod = sum % 11;
char check = check_code[mod];
if (check == id[17]) {
return 1; // 校验位正确
} else {
return 0; // 校验位错误
}
}
struct birth get_birthday_from_ID(char *ID) {
struct birth birthday;
char year_str[5], month_str[3], day_str[3];
memset(&birthday, 0, sizeof(struct birth));
memset(year_str, 0, sizeof(year_str));
memset(month_str, 0, sizeof(month_str));
memset(day_str, 0, sizeof(day_str));
strncpy(year_str, ID+6, 4);
strncpy(month_str, ID+10, 2);
strncpy(day_str, ID+12, 2);
birthday.year = atoi(year_str);
birthday.month = atoi(month_str);
birthday.day = atoi(day_str);
return birthday;
}
void get_all_person(struct person p[], int n) {
int i;
for (i = 0; i < n; i++) {
p[i].birthday = get_birthday_from_ID(p[i].ID);
p[i].flag = checkID(p[i].ID);
}
}
void display_person(struct person p[], int n, int flag) {
int i, count = 0;
for (i = 0; i < n; i++) {
if (p[i].flag == flag) {
printf("name: %s, ID: %s, birthday: %d-%d-%d\n", p[i].name, p[i].ID, p[i].birthday.year, p[i].birthday.month, p[i].birthday.day);
count++;
}
}
printf("Total number: %d\n", count);
}
int main() {
struct person p[4];
strcpy(p[0].ID, "123456197001010101");
strcpy(p[0].name, "张三");
strcpy(p[1].ID, "123456197001010102");
strcpy(p[1].name, "李四");
strcpy(p[2].ID, "123456197001010103");
strcpy(p[2].name, "王五");
strcpy(p[3].ID, "123456197001010104");
strcpy(p[3].name, "赵六");
get_all_person(p, 4);
printf("Correct IDs:\n");
display_person(p, 4, 1);
printf("\nIncorrect IDs:\n");
display_person(p, 4, 0);
return 0;
}
```
函数display_person接受一个person结构体数组p,整型n和整型flag,将输出每个公民的信息。在函数内部,使用for循环遍历结构体数组p中的每个元素,判断flag是否和当前元素的flag相同,如果相同则输出姓名、身份证号和出生年月。最后输出正确/错误的公民人数。
阅读全文
相关推荐
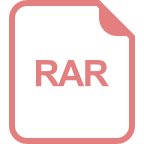
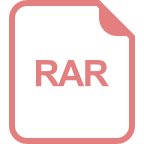
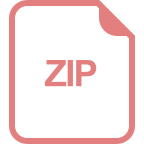
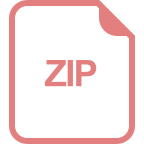
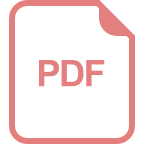
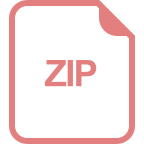
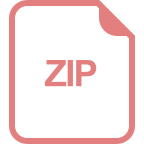
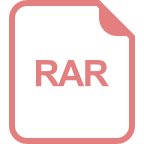
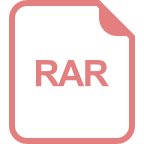
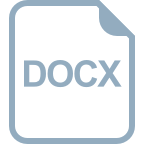
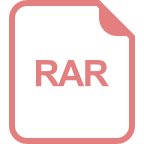
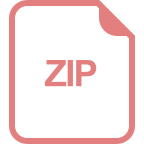
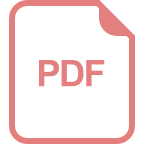
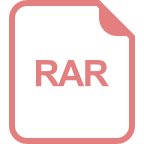
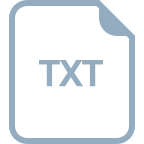
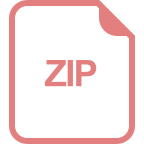
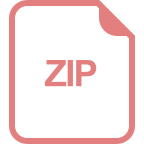
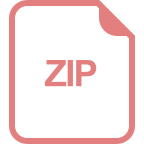