def __init__(self,player,groups): super().__init__(groups) self.sprite_type = 'weapon' direction = player.status.split('_')[0] # graphic full_path = f'../graphics/weapons/{player.weapon}/{direction}.png' self.image = pygame.image.load(full_path).convert_alpha() # placement if direction == 'right': self.rect = self.image.get_rect(midleft = player.rect.midright + pygame.math.Vector2(0,16)) elif direction == 'left': self.rect = self.image.get_rect(midright = player.rect.midleft + pygame.math.Vector2(0,16)) elif direction == 'down': self.rect = self.image.get_rect(midtop = player.rect.midbottom + pygame.math.Vector2(-10,0)) else: self.rect = self.image.get_rect(midbottom = player.rect.midtop + pygame.math.Vector2(-10,0))
时间: 2024-03-03 14:52:14 浏览: 125
这段代码是一个 Python 类的初始化函数,用来初始化一个武器精灵对象。它需要传入两个参数:一个是玩家对象,另一个是精灵组。这个函数首先调用父类的初始化函数,然后设置武器精灵的类型为 "weapon"。接着根据玩家对象的状态来确定武器的朝向,然后加载对应朝向的武器图片,并将其转换成 alpha 通道。最后根据不同的朝向,确定武器精灵的位置,并将其矩形赋值给 `self.rect` 属性。
相关问题
class Card(pygame.sprite.Sprite): def __init__(self,wg,pos,l): super().__init__() self.screen=wg.screen self.screen_rect=wg.screen.get_rect() self.settings=wg.settings self.formal_image=Formal_Img(l) self.image_front=f'images/card{random.randint(1,5)}.png' self.image_back=Player_Img(self.formal_image) self.image=self.image_front self.rect=self.image.get_rect() self.rect.center=pos self.is_flipped=False def flip(self): self.is_flipped=not self.is_flipped if self.is_flipped: self.image=self.image_back else: self.image=self.image_front
这是一个使用 Pygame 编写的 Card 类的构造函数和 flip() 方法。其中,Card 类继承了 Pygame 的 Sprite 类,并包含以下属性和方法:
属性:
- screen:表示游戏窗口的 surface 对象。
- screen_rect:表示游戏窗口的矩形对象。
- settings:表示游戏的设置。
- formal_image:表示卡片的正面图像。
- image_front:表示卡片的正面图像路径。
- image_back:表示卡片的背面图像。
- image:表示卡片当前显示的图像。
- rect:表示卡片的矩形对象,用于定位卡片在游戏窗口中的位置。
- is_flipped:表示卡片是否已翻转。
方法:
- flip():用于翻转卡片,即将卡片的正面图像和背面图像进行切换,并更新卡片的 is_flipped 属性。
class Tile(pygame.sprite.Sprite): def __init__(self,pos,groups,sprite_type,surface = pygame.Surface((TILESIZE,TILESIZE))): super().__init__(groups) self.sprite_type = sprite_type y_offset = HITBOX_OFFSET[sprite_type] self.image = surface if sprite_type == 'object': self.rect = self.image.get_rect(topleft = (pos[0],pos[1] - TILESIZE)) else: self.rect = self.image.get_rect(topleft = pos) self.hitbox = self.rect.inflate(0,y_offset)
这段代码是用 Python 编写的,使用了 Pygame 库来创建一个名为 Tile 的类。这个类继承了 Pygame.sprite.Sprite 类,用于在游戏中显示图像。
在 __init__ 方法中,传入了 pos、groups、sprite_type 和 surface 四个参数。其中,pos 表示 Tile 对象在游戏中的位置;groups 表示 Tile 对象所属的组;sprite_type 表示 Tile 对象的类型;surface 表示 Tile 对象的表面。
在方法中,首先调用了父类的 __init__ 方法进行初始化。然后根据传入的 sprite_type 和 HITBOX_OFFSET[sprite_type] 值,设置了 Tile 对象的 image、rect 和 hitbox 属性。
若 sprite_type 为 'object',则将 Tile 对象的 rect 设置为在 pos 坐标左上角位置的矩形,同时将 Tile 对象的 hitbox 属性上下扩展了 HITBOX_OFFSET[sprite_type] 个像素;否则,将 Tile 对象的 rect 直接设置为 pos 坐标的矩形,同时将 Tile 对象的 hitbox 属性上下扩展了 0 个像素。
阅读全文
相关推荐
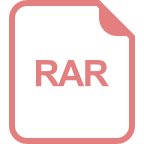
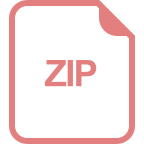
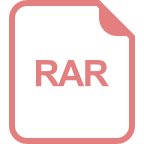











