def __init__(self, position): pygame.sprite.Sprite.__init__(self) self.image = pygame.image.load("素材/bullet_UK4.png").convert_alpha() self.rect = self.image.get_rect() self.rect.left, self.rect.top = position self.speed = 12 self.active = False self.mask = pygame.mask.from_surface(self.image) def move(self): self.rect.right += self.speed if self.rect.left >1023: self.active = False def reset(self, position): self.rect.left, self.rect.top = position self.active = True如何控制射速
时间: 2023-06-16 21:07:56 浏览: 287
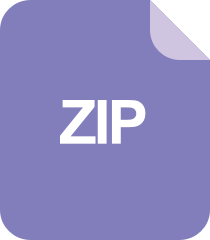
lib_base.zip_event_pygame.base_python graphic
要控制射速,可以通过两种方法实现:
1. 添加计时器,只有当计时器达到一定时间间隔后才能发射子弹。
例如,在类的初始化方法中添加以下属性:
```
self.last_shot = pygame.time.get_ticks()
self.shot_interval = 500 # 射击间隔为500毫秒
```
然后在发射子弹的方法中,判断当前时间与上一次发射子弹时间的时间差是否大于射击间隔,如果大于,则可以发射子弹并更新上一次发射子弹时间。
```
def fire(self):
current_time = pygame.time.get_ticks()
if current_time - self.last_shot > self.shot_interval:
# 发射子弹的代码
self.last_shot = current_time # 更新上一次发射子弹时间
```
这种方式可以精确控制射速,但需要额外的计时器代码。
2. 添加射速属性,限制一定时间内最多能发射多少个子弹。
例如,在类的初始化方法中添加以下属性:
```
self.shot_count = 0
self.max_shots = 3 # 在1秒内最多能发射3个子弹
self.shot_timer = pygame.time.get_ticks()
```
然后在发射子弹的方法中,判断当前时间与上一次发射子弹时间的时间差是否大于1秒,如果大于,则重置射速属性。
```
def fire(self):
current_time = pygame.time.get_ticks()
if current_time - self.shot_timer > 1000:
self.shot_timer = current_time
self.shot_count = 0
if self.shot_count < self.max_shots:
# 发射子弹的代码
self.shot_count += 1
```
这种方式可以简单地控制射速,但存在一定误差,因为无法精确控制每个子弹发射的时间间隔。
阅读全文
相关推荐
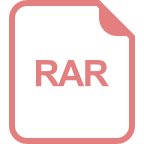















